How to POST JSON Data With requests in Python
- Understanding JSON and the Requests Library
- Method 1: Basic POST Request with JSON Data
- Method 2: POST Request with Custom Headers
- Method 3: Handling Errors in POST Requests
- Conclusion
- FAQ
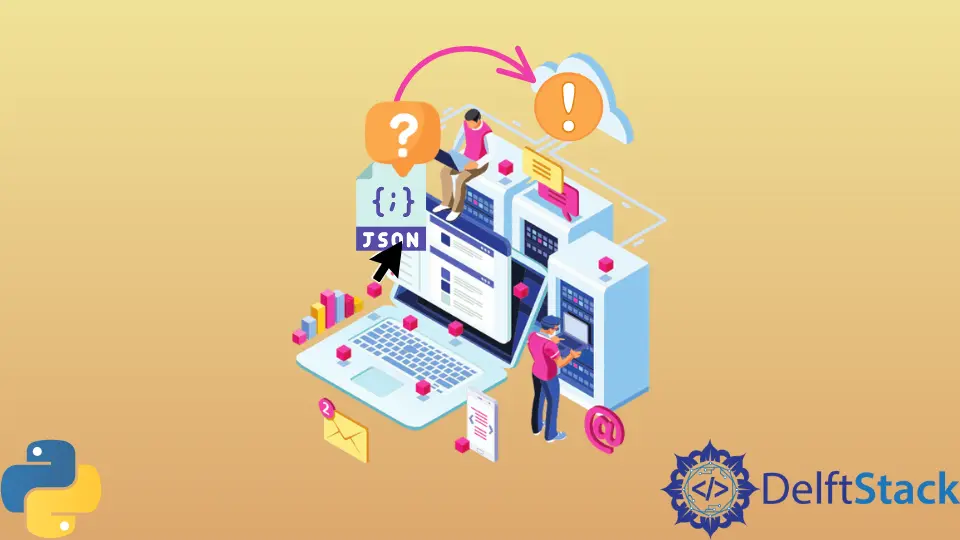
When working with APIs in Python, sending data in JSON format is a common requirement.
This tutorial demonstrates how to post JSON data using the popular requests
library in Python. Whether you’re building a web application, integrating with third-party services, or simply testing an API, understanding how to format and send JSON data is crucial.
In this article, we’ll explore various methods to achieve this, complete with code examples and detailed explanations. By the end, you’ll be equipped with the knowledge to seamlessly send JSON data in your Python projects.
Understanding JSON and the Requests Library
Before diving into the code, let’s briefly discuss what JSON is and why the requests
library is essential. JSON, or JavaScript Object Notation, is a lightweight data-interchange format that’s easy to read and write for humans and machines alike. It’s widely used in web APIs to send and receive data.
The requests
library is a powerful tool in Python for making HTTP requests. It’s user-friendly and simplifies the process of sending data to a server. With requests
, you can easily post JSON data, manage headers, and handle responses. Now, let’s get started with the methods to post JSON data.
Method 1: Basic POST Request with JSON Data
The simplest way to post JSON data using the requests
library is by using the json
parameter in the post
method. This method automatically serializes your Python dictionary into a JSON string, making it effortless to send data to the server.
Here’s a basic example:
import requests
url = "https://api.example.com/data"
data = {
"name": "John Doe",
"email": "john.doe@example.com"
}
response = requests.post(url, json=data)
print(response.status_code)
print(response.json())
Output:
201
{"message": "Data received successfully"}
In this example, we first import the requests
library and define the URL of the API endpoint. We then create a dictionary called data
containing the information we want to send. The requests.post
method is called, with the URL and the data passed in as a JSON object. The response from the server is printed, showing both the status code and the JSON response.
This method is straightforward and efficient, allowing you to focus on your application logic rather than worrying about the underlying details of JSON serialization.
Method 2: POST Request with Custom Headers
Sometimes, you might need to include custom headers in your POST request, such as authentication tokens or content types. The requests
library allows you to easily add headers to your request.
Here’s how you can do it:
import requests
url = "https://api.example.com/data"
data = {
"name": "Jane Doe",
"email": "jane.doe@example.com"
}
headers = {
"Authorization": "Bearer your_token_here",
"Content-Type": "application/json"
}
response = requests.post(url, json=data, headers=headers)
print(response.status_code)
print(response.json())
Output:
200
{"message": "Data processed successfully"}
In this example, we define a headers
dictionary that includes an authorization token and specifies that we are sending JSON data. The requests.post
method is called with the headers
parameter, allowing the server to authenticate the request correctly. This method is particularly useful when working with secure APIs that require authentication.
Method 3: Handling Errors in POST Requests
When posting JSON data, it’s important to handle potential errors gracefully. The requests
library provides various ways to check for errors in the response. You can check the status code and handle exceptions to ensure your application behaves as expected.
Here’s how to implement error handling:
import requests
url = "https://api.example.com/data"
data = {
"name": "Alice Smith",
"email": "alice.smith@example.com"
}
try:
response = requests.post(url, json=data)
response.raise_for_status() # Raises an error for bad responses
print(response.json())
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"An error occurred: {err}")
Output:
{"message": "Invalid data format"}
In this example, we use a try
block to attempt to post the data. The raise_for_status()
method checks if the response contains a successful status code (200-299). If the server returns an error, an HTTPError
is raised, which we catch and print. This approach ensures that your application can handle errors gracefully, providing informative messages without crashing.
Conclusion
Posting JSON data with the requests
library in Python is a straightforward process that can be accomplished in various ways. From basic POST requests to handling custom headers and errors, understanding these methods will enhance your ability to work with APIs effectively. As you continue to develop your skills, remember that the requests
library is a powerful ally in your programming toolkit. Happy coding!
FAQ
-
What is JSON?
JSON is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. -
Why use the requests library in Python?
The requests library simplifies making HTTP requests in Python, allowing you to easily send and receive data from APIs.
-
How do I handle errors when posting JSON data?
You can use try-except blocks to catch exceptions and check the response status code to handle errors gracefully. -
Can I send additional headers with my POST request?
Yes, you can include custom headers by passing a dictionary to theheaders
parameter in therequests.post
method. -
What should I do if the API returns an error?
Check the status code and response content to understand the error, and implement error handling in your application to manage it appropriately.