How to Use Pi in Python
-
Use the
math.pi()
Function to Get the Pi Value in Python -
Use the
numpy.pi()
Function to Get the Pi Value in Python -
Use the
scipy.pi()
Function to Get the Pi Value in Python -
Use the
math.radians()
Function to Get the Pi Value in Python
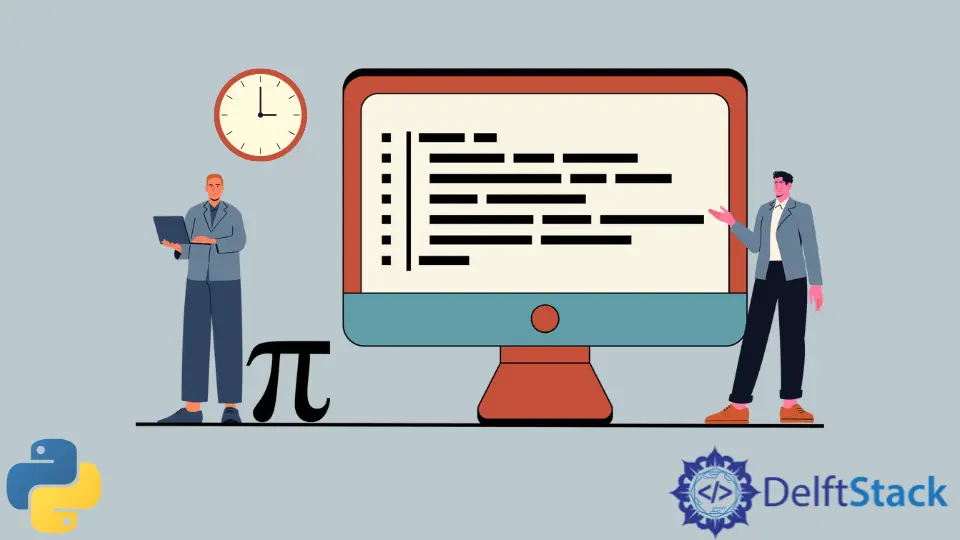
Python has a lot of objects and modules available for mathematical and scientific calculation.
In this tutorial, we will find and use the pi value in Python.
Use the math.pi()
Function to Get the Pi Value in Python
For this, we will use the math
module. The math
module provides access to the mathematical functions in the Python programming language.
There are a lot of functions associated with this module. The math.pi()
function is used for accessing the value of pi in Python. First of all, import the math
module to access the pi
function.
For example,
import math
math.pi
Output:
3.141592653589793
We can now use this value for our calculations and expressions.
Use the numpy.pi()
Function to Get the Pi Value in Python
The numpy.pi()
can also return the value of pi in Python.
For example,
import numpy
print(numpy.pi)
Output:
3.141592653589793
Use the scipy.pi()
Function to Get the Pi Value in Python
The pi()
function from the scipy
module can also return the value of pi.
import scipy
print(scipy.pi)
Output:
3.141592653589793
All three modules return the same value. The only reason why this function exists in three modules is that it allows us to work with pi value without importing any other module. For example, while working with NumPy
, we won’t have to import math
or scipy
to get the value of pi.
Use the math.radians()
Function to Get the Pi Value in Python
This is an unconventional method and is hardly ever used. There is another way to convert degrees to radians in Python without dealing with pi directly for the particular case. There is a function named radians()
converting degree to radians in the math
module.
import math
math.radians(90)
Output:
1.5707963267948966
We can use this function to get the value of pi, as shown below.
import math
math.radians(180)
Output:
3.141592653589793
As you can see, when we convert 180 degrees to radians, we get the value for pi.