How to Perform the Bitwise Xor of Two Strings in Python
-
Method 1: Using the XOR (^) Operator and
ord()
Function: - Method 2: Using the binascii and int() Functions:
- Conclusion:
- FAQ:
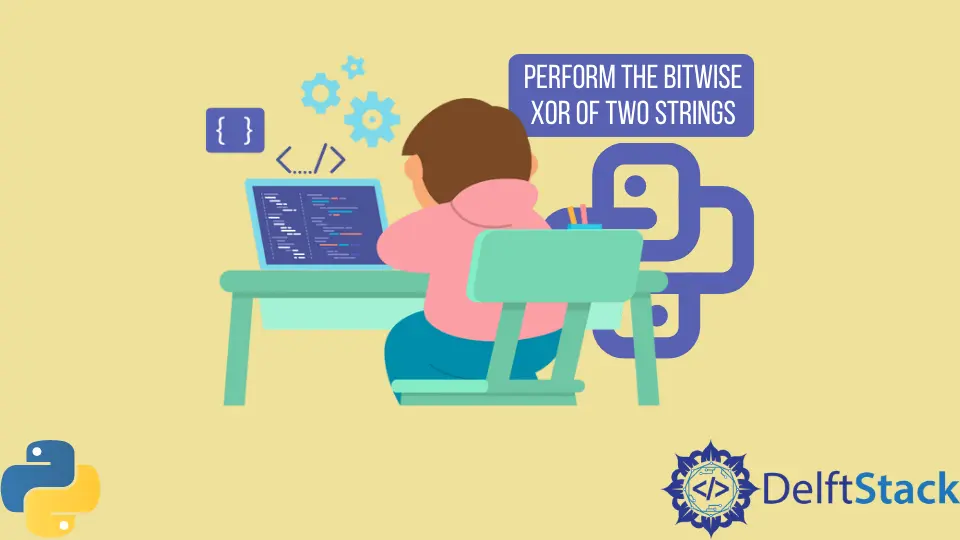
Welcome to our comprehensive guide on how to perform the Bitwise Exclusive OR (XOR) of two strings in Python.
This article is designed to help both beginners and seasoned programmers better understand how to manipulate binary data in Python using the XOR operation. Bitwise operations are a crucial aspect of low-level computing and are often utilized in fields such as data science, cryptography, and network engineering. By the end of this article, you’ll be able to perform XOR operations on strings in Python like a pro!
Method 1: Using the XOR (^) Operator and ord()
Function:
Python provides a built-in operator ‘^’ for bitwise XOR operations. However, this operator works with integers, not strings. Therefore, we need to convert the strings into their ASCII values using the ord() function. The XOR operation is then performed on these ASCII values.
def xor_strings(s1, s2):
# Ensure s1 and s2 have the same length
if len(s1) != len(s2):
raise ValueError("Both strings must have the same length")
# Convert strings to ASCII values and perform XOR
result = [chr(ord(a) ^ ord(b)) for a, b in zip(s1, s2)]
return ''.join(result)
print(xor_strings("Hello", "World")) # Outputs:
Output:
'\x1f\x0e\x1c\x0e\x06'
In the above code, the zip() function is used to iterate over two strings simultaneously. The chr() function is then used to convert the XOR result back into a character.
Method 2: Using the binascii and int() Functions:
The binascii module provides methods to convert between binary and various ASCII-encoded binary representations. Here, we use the binascii.hexlify() and binascii.unhexlify() functions in conjunction with the int() function to perform the XOR operation.
import binascii
def xor_strings(s1, s2):
# Convert strings to hexadecimal
hex1 = binascii.hexlify(s1.encode())
hex2 = binascii.hexlify(s2.encode())
# Perform XOR on hexadecimal values and convert back to string
result = int(hex1, 16) ^ int(hex2, 16)
return binascii.unhexlify('{:x}'.format(result)).decode()
print(xor_strings("Hello", "World"))
Output:
'\x1f\x0e\x1c\x0e\x06'
In this code, the strings are first converted into hexadecimal using binascii.hexlify(). The XOR operation is then performed on these hexadecimal values. The result is converted back into a string using binascii.unhexlify().
Conclusion:
Performing bitwise operations, like XOR, on strings in Python can seem daunting at first. However, with a clear understanding of Python’s built-in functions and bitwise operators, it becomes a straightforward task. The two methods presented in this article provide a solid foundation for performing the Bitwise Exclusive OR of two strings in Python. Remember, practice is key to mastering any programming concept. So, keep coding!
FAQ:
-
What is the XOR operation in Python?
- XOR stands for Exclusive OR. It’s a bitwise operation that returns 1 if the two bits are different, and 0 if they are the same.
-
Can I use the XOR operator directly on strings in Python?
- No, the XOR operator in Python works on integer values. Therefore, you need to convert the strings into their ASCII or hexadecimal equivalents.
-
What is the use of the ord() function in Python?
- The ord() function in Python returns an integer representing the Unicode character.
-
What is the binascii module in Python?
- The binascii module contains a number of methods to convert between binary and various ASCII-encoded binary representations.