Package vs Module in Python
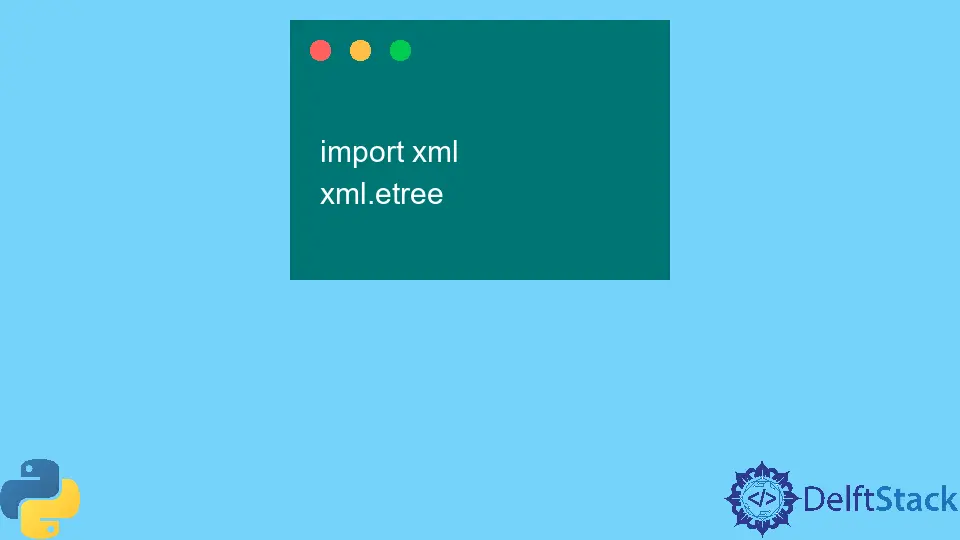
We have different packages available in Python. Each package has its area of focus. While working with Python, we use different functionalities belonging to different modules or packages. We use the terms modules and packages interchangeably.
We will understand the difference between these two terms in this article.
A module is a script file consisting of various functions and global variables. The file is saved with a .py
extension. These files are executable and can store different functions and objects. To organize modules, we have the concept of Packages in Python.
On the other hand, a package is a simple directory consisting of collections of modules. A package contains an additional __init__.py
file so that the interpreter interprets it as a package. We can interpret it as a hierarchical file directory structure that defines a single application environment. A package may be composed of further smaller sub-packages.
One should also note the similarities between the two. To use them, we import them using the import
statement. This creates an object of type module
regardless of whether we are importing a module or package. However, on importing a package, only the classes, functions, variables are visible, which are directly visible in the __init__.py
file.
Let us understand this with an example.
import xml
xml.etree
The above code returns an AttributeError
. To overcome this, we can consider the code given below.
import xml.etree
xml.etree
The above code will not give any errors. Hopefully, by now, the difference between a package and a module is clear.
Related Article - Python Module
- How to Import Files in Python
- Type Hints in Python
- The fnmatch Module in Python
- The Telnetlib Module in Python
- Socket Programming in Python: A Beginners Guide
- How to Import All Modules in One Directory in Python