How to Use the waitKey Function in OpenCV
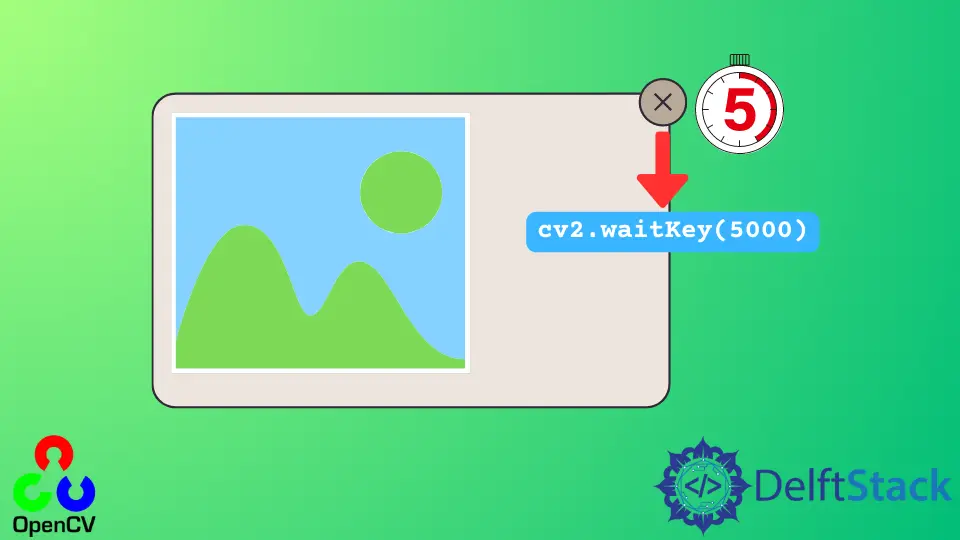
This tutorial will discuss closing an image window using the waitKey()
function of OpenCV.
Using the waitKey()
Function in OpenCV
The waitKey()
function in OpenCV is used to wait for a specific time interval and then close the active image window.
We can pass the delay in milliseconds inside the waitKey()
function, and the function will wait for that specific time, and then it will close the active image window.
If we press a key from the keyboard while the image window is active, the window will close.
For example, consider we want the image to close automatically after 5 seconds; it will close after 5 seconds if we don’t press any key from the keyboard. Still, if we press a key from the keyboard before 5 seconds, the window will close.
If we don’t want to wait for a specific time and want to close the image when a key is pressed, we have to pass 0 or nothing inside the waitKey()
function. In this case, the function will wait for the key, and when a key is pressed, it will close the window.
See the example code below.
import cv2
saved_image = cv2.imread("Image_name.jpg")
cv2.imshow("image", saved_image)
cv2.waitKey(5000)
cv2.destroyAllWindows()
The above code will wait for five seconds, and then it will close the window, and if a key is present at that time, the window will close. Note that we have to use the destroyAllWindows()
function for the waitKey()
function to work properly in the Jupyter
Notebook.
The destroyAllWindows()
function will close all the open windows. But if we are in some other software like PyCharm
, we don’t have to use the destroyAllWindows()
function.