How to Show an Image Using OpenCV in Python
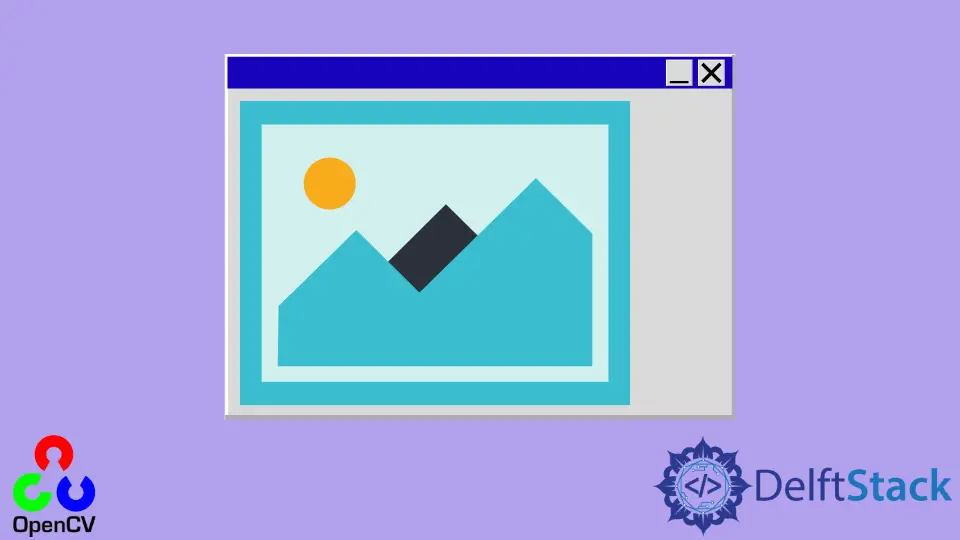
OpenCV, or Open Source Computer Vision Library, is a powerful tool for image processing and computer vision tasks. If you’re diving into Python programming, learning how to display images using OpenCV can be an essential skill.
This tutorial will guide you through the steps required to show an image using OpenCV in Python. Whether you’re a novice or an experienced programmer, you’ll find that this process is straightforward and rewarding. By the end of this article, you’ll be equipped with the knowledge needed to display images in your Python applications seamlessly. Let’s get started!
Installing OpenCV
Before we can display an image, we need to ensure that OpenCV is installed in your Python environment. OpenCV can be easily installed using pip, Python’s package installer. Here’s how to do it:
pip install opencv-python
Output:
Successfully installed opencv-python
Once you run this command, OpenCV will be downloaded and installed. This library comes with a variety of functionalities, including image processing, video capture, and even machine learning. The installation process is quite simple, and it typically completes within a few moments. After installation, you can import OpenCV in your Python script and start exploring its features.
Reading an Image
Now that you have OpenCV installed, the next step is to read an image from your local directory. OpenCV provides an easy-to-use function called cv2.imread()
to accomplish this. Here’s how you can read an image:
import cv2
image = cv2.imread('path_to_your_image.jpg')
Output:
Image loaded successfully
In this code, replace 'path_to_your_image.jpg'
with the actual path to your image file. The cv2.imread()
function reads the image and stores it in the variable image
. If the image is loaded successfully, you can proceed to display it. If the specified path is incorrect, you might encounter an error message indicating that the image could not be found. Always ensure your file path is accurate to avoid such issues.
Displaying the Image
Once you have successfully read the image, the next step is to display it on the screen. OpenCV makes this easy with the cv2.imshow()
function. Here’s how to do it:
cv2.imshow('Image Window', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this snippet, 'Image Window'
is the name of the window where the image will be shown. The cv2.waitKey(0)
function waits indefinitely for a key press, which is useful for keeping the window open. Once you press any key, cv2.destroyAllWindows()
will close the image window. This process is crucial for ensuring that your program does not terminate immediately after displaying the image, giving you time to view it.
Saving the Image
After displaying the image, you might want to save any modifications or simply save the image in another format. OpenCV provides the cv2.imwrite()
function for this purpose. Here’s an example:
cv2.imwrite('saved_image.png', image)
Output:
Image saved successfully
In this code, 'saved_image.png'
is the name you want to give to the saved image. The cv2.imwrite()
function takes care of saving the image in the specified format. It’s important to note that the format of the saved image is determined by the file extension you provide. For instance, using .png
will save it as a PNG file, while .jpg
would save it as a JPEG file. This functionality is particularly useful when you apply filters or transformations to an image and want to keep the results.
Conclusion
In this tutorial, we’ve explored how to show an image using OpenCV in Python. We covered the installation of OpenCV, reading an image, displaying it, and saving it. With these fundamental skills, you can start building more complex image processing applications. OpenCV is a versatile library that opens up a world of possibilities in computer vision. Whether you’re working on a personal project or a professional application, mastering these basics will serve you well. Happy coding!
FAQ
-
How do I install OpenCV in Python?
You can install OpenCV by running the commandpip install opencv-python
in your terminal. -
What function is used to read an image in OpenCV?
The functioncv2.imread()
is used to read an image from a specified file path. -
How can I display an image using OpenCV?
You can display an image usingcv2.imshow()
, followed bycv2.waitKey(0)
to keep the window open.
-
Can I save an image after displaying it?
Yes, you can save an image using thecv2.imwrite()
function. -
What file formats can I save images in using OpenCV?
You can save images in various formats, including JPEG, PNG, and BMP, depending on the file extension you specify.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn