How to OpenCV Save Image
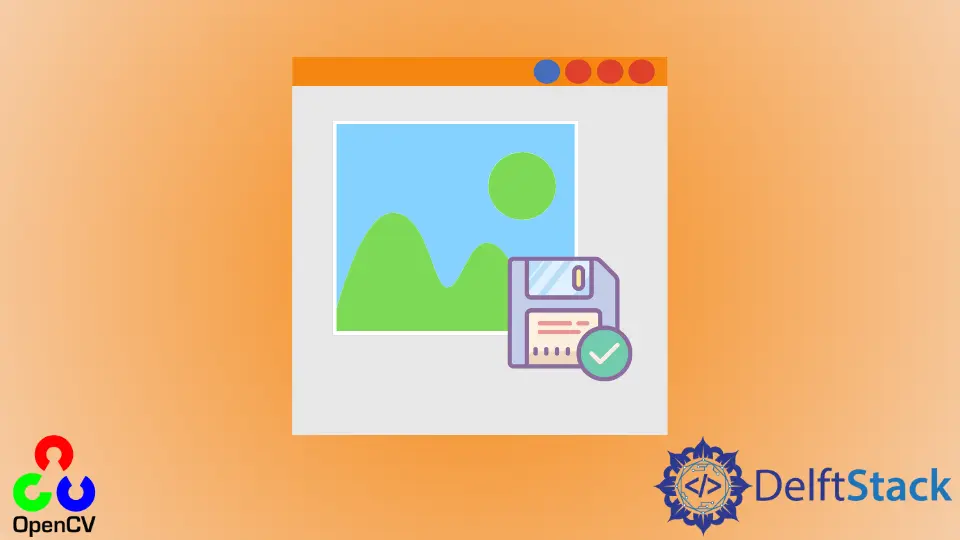
This tutorial will discuss saving an image using the imwrite()
function of OpenCV.
Save Images in OpenCV
An image is composed of pixels that are placed in a matrix. Each pixel contains a color value as a BGR triplet.
For example, a 512-by-512 image is composed of 512 columns and 512 rows, and the total number of pixels present in the image is equal to the number of rows multiplied by the number of columns. A BGR triplet value contains three values that range from 0 to 255 in an 8-bit image.
The first value corresponds to the intensity of the blue color. For example, a pixel of value (255,0,0) will have a dark blue color, and a pixel of value (50,0,0) will have a light blue color.
The second and third BRG triplet values correspond to green and red colors. We can set the value of these three colors to make any color.
In an 8-bit image, a single pixel can have 256 multiplied by 256 multiplied by 255 different colors. We can use the imwrite()
function of OpenCV to save an image with our desired image format.
For example, let’s create a color image and save it as jpg
. See the code below.
import cv2
import numpy as np
img = np.zeros((512, 512, 3), dtype=np.uint8)
img[:, 0:250] = (255, 255, 0)
img[:, 250:512] = (0, 255, 255)
cv2.imwrite("Image_name.jpg", img)
saved_image = cv2.imread("Image_name.jpg")
cv2.imshow("image", saved_image)
cv2.waitKey()
Output:
We used the zeros()
function of NumPy
to create the above image. The first argument of the imwrite()
function is the name and extension of the file, and the second argument is the variable in which the image is saved.
In the above code, we used the imread()
function to read the image that we saved and displayed it using the imshow()
function of OpenCV. The image that we want to save should be 8 bit and have one or three channels.
In the case of a 16-bit image, we can only save it in the TIFF, JPEG, and PNG format. In the case of a 32-bit image, we can save it in TIFF, HDR, and OpenEXR
format.
Also, the image should be in BGR color space. If not, we can use the cvtColor()
function of OpenCV to change one color space into another.
If the channel order or depth is different, we can use the convertTo()
function to convert the image to a supported format.
We can also save an 8 or 16-bit BGRA color space image using the above function.