How to Normalize Images in Python OpenCV
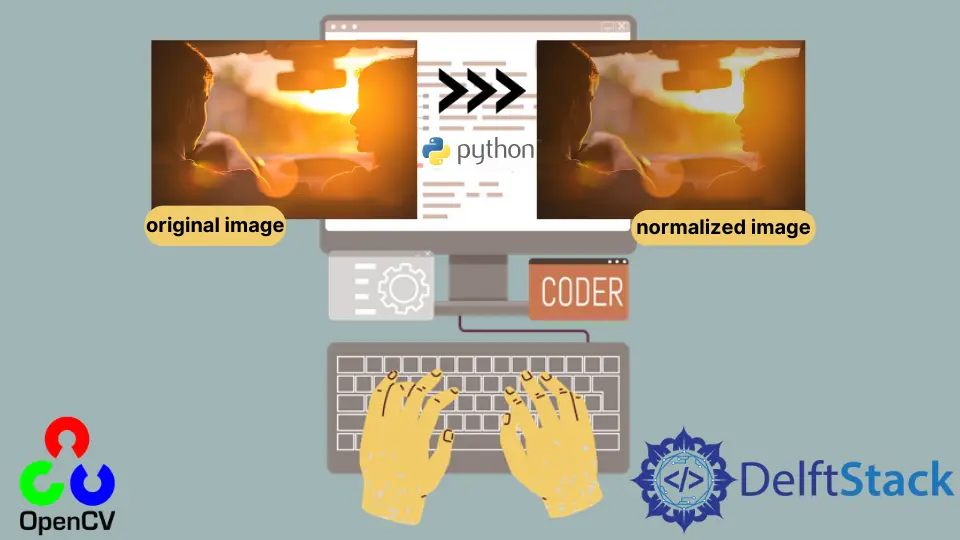
This tutorial will discuss normalizing an image using the normalize()
function of OpenCV in Python.
Use the normalize()
Function of OpenCV to Normalize an Image in Python
Normalization in image processing is used to change the intensity level of pixels. It is used to get better contrast in images with poor contrast due to glare.
We can use the normalize()
function of OpenCV to normalize an image. The normalize()
function’s first argument is the source image that we want to normalize.
The second argument is the destination image, creating an output image with our desired dimensions or size. The third argument is the lower value of range in which we want to normalize an image.
The fourth argument is the upper value of the range in which we want to normalize an image. The fifth argument is the type of normalization like cv2.NORM_INF
, cv2.NORM_L1
, and cv2.NORM_MINMAX
.
Every normalization type uses its formula to calculate the normalization. The sixth argument is used to set the data type of the output image.
The seventh argument is used to create a mask, and it is useful when we don’t want to normalize the whole image. Instead, we only want to normalize a portion of the image.
We can define that portion in the mask so that normalization will only be performed on the masked portion.
For example, let’s reduce the glare present in an image using the normalize()
function. See the code below.
import cv2
import numpy as np
image = cv2.imread("glare2.jpg")
image_norm = cv2.normalize(image, None, alpha=0, beta=200, norm_type=cv2.NORM_MINMAX)
cv2.imshow("original Image", image)
cv2.imshow("Normalized Image", image_norm)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
We can change the arguments and the normalization type according to the given image to get the desired output.
By default, the alpha value is 1, and the beta value is 0. By default, the type of normalization is set to cv2.NORM_L2
. If we don’t define the values for these arguments, the function will use the default values.