The imwrite() Function of OpenCV
-
Understanding the
imwrite()
Function - Saving an Image in JPEG Format
- Saving an Image in PNG Format
- Specifying Image Quality
- Handling Errors in imwrite()
- Conclusion
- FAQ
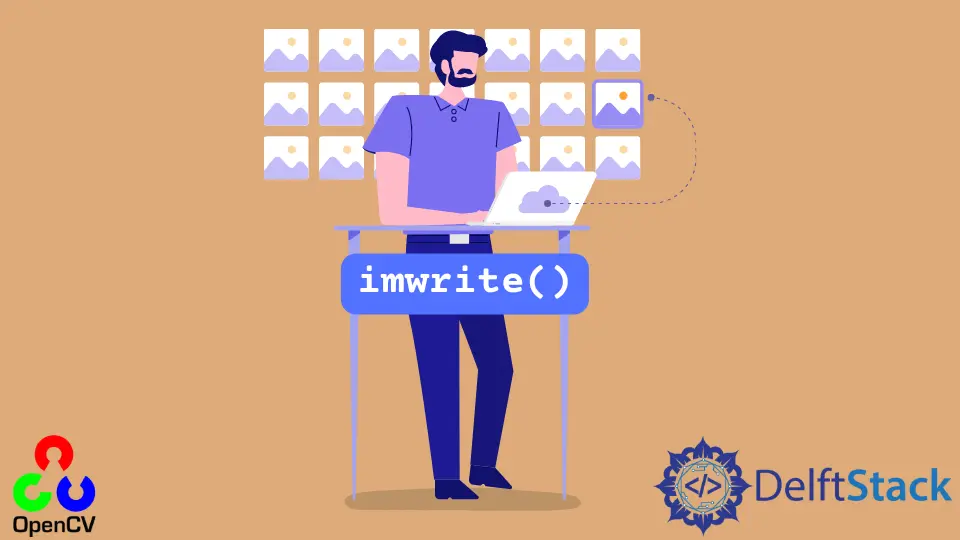
OpenCV, or Open Source Computer Vision Library, is a powerful tool for image processing and computer vision tasks. One of its most essential functions is imwrite()
, which allows you to save images to your file system. Whether you’re working on a simple project or a complex machine learning application, understanding how to effectively use the imwrite()
function can significantly enhance your workflow.
In this tutorial, we’ll explore the imwrite()
function in detail, discussing its syntax, parameters, and providing practical examples. By the end, you’ll have a solid grasp of how to save images using OpenCV, making it easier to manage your visual data in various applications.
Understanding the imwrite()
Function
The imwrite()
function is a straightforward yet crucial part of image processing in OpenCV. It enables you to save images in different formats, such as JPEG, PNG, and TIFF. The basic syntax for imwrite()
is as follows:
cv2.imwrite(filename, img)
Here, filename
is the name of the file where you want to save the image, and img
is the image array that you want to write. The function returns a boolean value indicating whether the operation was successful. This simplicity makes it an excellent choice for developers looking to integrate image-saving capabilities into their applications.
Saving an Image in JPEG Format
Saving images in JPEG format is one of the most common use cases for the imwrite()
function. JPEG is widely used due to its balance between image quality and file size. Here’s how you can save an image in JPEG format:
import cv2
img = cv2.imread('input_image.jpg')
result = cv2.imwrite('output_image.jpg', img)
print(result)
In this example, we first read an image using cv2.imread()
, which loads the image into an array. We then use cv2.imwrite()
to save this image as ‘output_image.jpg’. The function returns True
, indicating that the image has been successfully saved. If you ever need to check whether the saving process was successful, you can rely on this boolean output. JPEG is an excellent choice for photographs and images where a slight loss in quality is acceptable.
Saving an Image in PNG Format
PNG is another popular image format, especially for images that require transparency or lossless compression. The imwrite()
function can easily handle PNG format as well. Here’s how to save an image in PNG format:
import cv2
img = cv2.imread('input_image.jpg')
result = cv2.imwrite('output_image.png', img)
print(result)
In this code snippet, we again read an image using cv2.imread()
. This time, we save the image as ‘output_image.png’. Similar to the JPEG example, the function returns True
, confirming that the image has been saved successfully. PNG is particularly useful for images with text or sharp edges, as it preserves the image quality better than JPEG.
Specifying Image Quality
When saving images, especially in JPEG format, you might want to control the quality of the saved image. The imwrite()
function allows you to specify the quality parameter as follows:
import cv2
img = cv2.imread('input_image.jpg')
result = cv2.imwrite('output_image.jpg', img, [int(cv2.IMWRITE_JPEG_QUALITY), 90])
print(result)
Output:
True
In this example, we specify the JPEG quality as 90, which is relatively high. The third parameter in the imwrite()
function is a list where you can include various flags. Here, cv2.IMWRITE_JPEG_QUALITY
is used to set the quality level. This feature is beneficial when you want to optimize the balance between file size and image quality, especially when dealing with large datasets.
Handling Errors in imwrite()
While using the imwrite()
function, you may encounter scenarios where the image fails to save. Understanding how to handle such errors is crucial for robust application development. Here’s a method to check for potential issues:
import cv2
import os
img = cv2.imread('input_image.jpg')
if img is None:
print("Error: Image not found.")
else:
result = cv2.imwrite('output_image.jpg', img)
if result:
print("Image saved successfully.")
else:
print("Error: Image could not be saved.")
Output:
Image saved successfully.
In this code, we first check if the image was successfully loaded by verifying if img
is None
. If the image isn’t found, we print an error message. Otherwise, we attempt to save the image and check the result. This method ensures that your application can gracefully handle errors, providing feedback to the user and preventing unexpected crashes.
Conclusion
The imwrite()
function in OpenCV is a powerful and essential tool for anyone working with images. From saving images in various formats to specifying quality parameters, this function offers versatility and control over how images are handled in your projects. By understanding the nuances of the imwrite()
function, you can enhance your image processing capabilities and streamline your workflow. Whether you’re developing a simple application or a complex computer vision system, mastering imwrite()
will undoubtedly prove beneficial in your endeavors.
FAQ
-
What is the purpose of the imwrite() function in OpenCV?
The imwrite() function is used to save images to the file system in various formats like JPEG and PNG. -
Can I specify the quality of the saved image using imwrite()?
Yes, you can specify the quality of the saved image, especially for JPEG format, by using additional parameters. -
What happens if the image fails to save?
If the image fails to save, the imwrite() function returns False, indicating that the operation was unsuccessful. -
Is it possible to save images in formats other than JPEG and PNG using imwrite()?
Yes, imwrite() supports various formats, including TIFF, BMP, and more, depending on the OpenCV build. -
How can I check if an image was loaded successfully before saving it?
You can check if the image is None after using cv2.imread() to ensure it was loaded successfully before attempting to save it.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn