OpenCV-contrib Module in Python
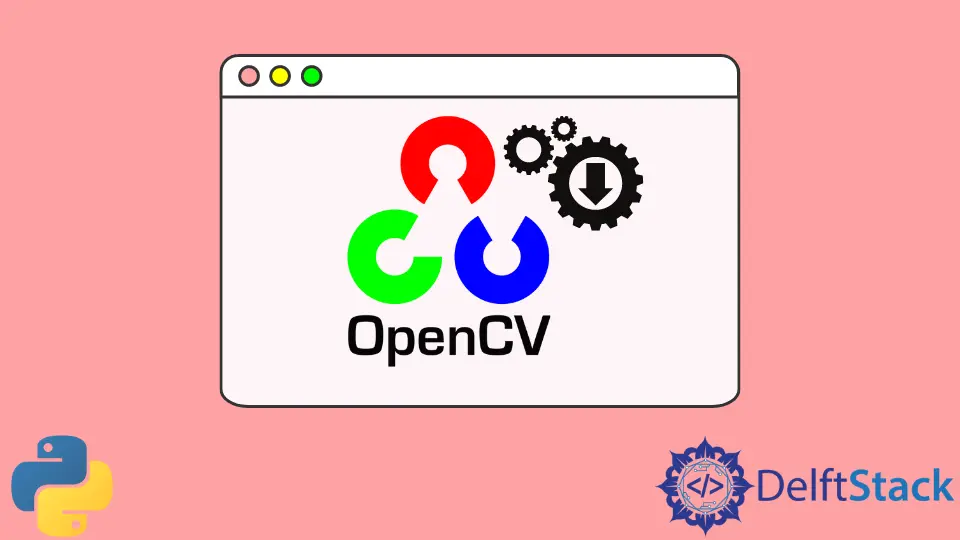
OpenCV is a powerful library for computer vision tasks, and its contrib module extends its capabilities even further. If you’re looking to enhance your Python projects with advanced computer vision functionalities, the OpenCV-contrib library is a must-have.
This tutorial will guide you through the process of using OpenCV-contrib in Python, from installation to practical applications. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to leverage the full potential of OpenCV-contrib. Let’s dive in and discover how to get started!
What is OpenCV-contrib?
OpenCV-contrib is an additional module for OpenCV that provides extra functionalities and algorithms not included in the main library. It contains experimental and non-free modules, which can be incredibly useful for advanced computer vision tasks. The contrib module includes features like tracking, machine learning, and image processing that can significantly enhance your projects.
To use OpenCV-contrib, you first need to install it alongside the main OpenCV library. This tutorial will walk you through the installation process and show you how to utilize some of the most popular features available in the contrib module.
Installing OpenCV-contrib
Before you can start using OpenCV-contrib in Python, you need to install it. Here’s how to do it using Git. First, ensure you have Git installed on your machine. If you haven’t done so, you can download it from the official Git website.
-
Clone the OpenCV repository:
git clone https://github.com/opencv/opencv.git
-
Clone the OpenCV-contrib repository:
git clone https://github.com/opencv/opencv_contrib.git
-
Navigate to the OpenCV directory:
cd opencv
-
Create a build directory:
mkdir build cd build
-
Configure the build with CMake, linking the contrib modules:
cmake -DOPENCV_EXTRA_MODULES_PATH=../opencv_contrib/modules ..
-
Compile the library:
make -j$(nproc)
-
Finally, install OpenCV:
sudo make install
This process will install both OpenCV and the contrib module, allowing you to access the extended features in your Python projects.
Using OpenCV-contrib in Python
Once you’ve installed OpenCV-contrib, it’s time to start using it in your Python projects. Below, we’ll explore some practical examples of how to utilize the functionalities provided by the contrib module.
Example 1: Implementing Structured Forests for Fast Edge Detection
One of the valuable features of the OpenCV-contrib module is the structured forests for fast edge detection. This technique is particularly useful in scenarios where you need to quickly identify edges in images.
Here’s how you can implement it:
import cv2
import numpy as np
image = cv2.imread('image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Load pre-trained model
model = cv2.ximgproc.createStructuredEdgeDetection("model.yml")
# Detect edges
edges = model.detectEdges(gray)
# Display results
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, we first load an image and convert it to grayscale. Then, we utilize the createStructuredEdgeDetection
function to load a pre-trained model for edge detection. The detectEdges
function processes the grayscale image, and the resulting edges are displayed in a window. This method is efficient and can be applied to various images for quick edge detection.
Example 2: Implementing Object Tracking with MOSSE
Another exciting feature in OpenCV-contrib is the MOSSE (Minimum Output Sum of Squared Error) tracker, which is used for object tracking. This tracker is fast and effective, making it suitable for real-time applications.
Here’s how to implement object tracking using MOSSE:
import cv2
cap = cv2.VideoCapture(0)
ret, frame = cap.read()
# Select ROI for tracking
bbox = cv2.selectROI(frame, False)
tracker = cv2.TrackerMOSSE_create()
# Initialize tracker
tracker.init(frame, bbox)
while True:
ret, frame = cap.read()
success, bbox = tracker.update(frame)
if success:
(x, y, w, h) = [int(v) for v in bbox]
cv2.rectangle(frame, (x, y), (x + w, y + h), (255, 0, 0), 2)
cv2.imshow('Tracking', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
In this example, we first capture video from the webcam and allow the user to select a region of interest (ROI) for tracking. The MOSSE tracker is initialized with the selected ROI. In the loop, we update the tracker with each new frame and draw a rectangle around the tracked object. The result is a real-time video feed displaying the tracked object, showcasing the effectiveness of the MOSSE tracker.
Conclusion
The OpenCV-contrib module is a powerful extension of the OpenCV library that offers a wealth of additional functionality for computer vision tasks. From advanced edge detection techniques to efficient object tracking, the contrib module opens up new possibilities for developers and researchers alike. By following this tutorial, you now have the foundational knowledge to install and utilize OpenCV-contrib in your Python projects. As you explore its features, you’ll find countless ways to enhance your applications and push the boundaries of what’s possible in computer vision.
FAQ
-
What is the OpenCV-contrib module?
The OpenCV-contrib module is an extension of the OpenCV library that includes additional algorithms and functionalities not found in the main library. -
How do I install OpenCV-contrib?
You can install OpenCV-contrib by cloning the repositories from GitHub, configuring with CMake, and compiling the library. -
What are some features of OpenCV-contrib?
OpenCV-contrib includes features like advanced tracking algorithms, machine learning models, and enhanced image processing techniques. -
Can I use OpenCV-contrib for real-time applications?
Yes, many features in OpenCV-contrib, such as the MOSSE tracker, are optimized for real-time performance. -
Is OpenCV-contrib free to use?
Yes, OpenCV and the contrib module are open-source and free to use under the Apache 2.0 license.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn