One-Line for Loop in Python
-
a Simple One-Line
for
Loop in Python -
List Comprehension in Python Using the One-Line
for
Loop -
List Comprehension in Python With the
if ... else
Statement Using the One-Linefor
Loop - Conclusion
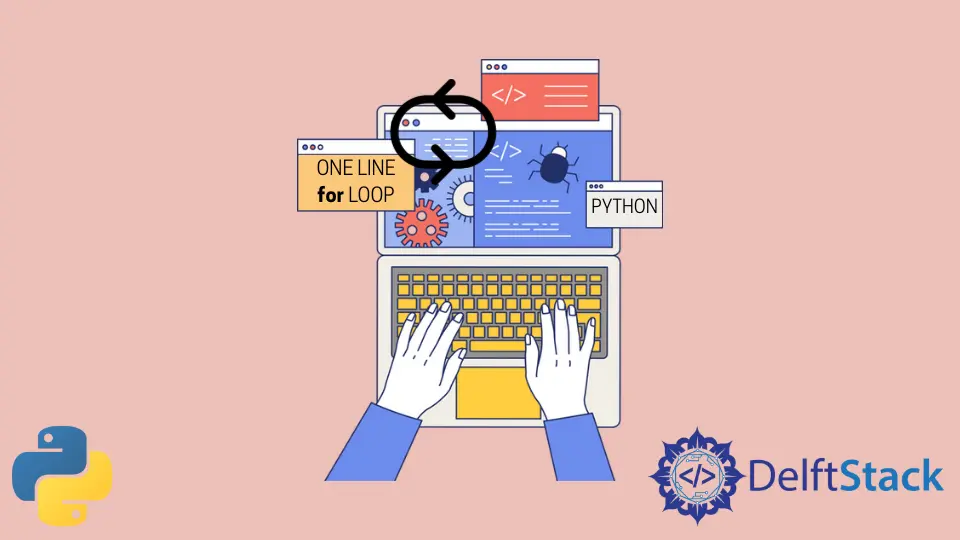
This tutorial will demonstrate various methods to implement a one-line for
loop in Python. One-line for
loops can take various forms, such as iterating through iterable objects or sequences, as well as using list comprehensions and list comprehensions with if ... else
statements.
a Simple One-Line for
Loop in Python
A simple one-line for
loop is the basic for
loop that iterates through a sequence or an iterable object. You can use either an iterable object with the for
loop or the range()
function, and the iterable object can be a list, array, set, or dictionary.
Let’s start with an example of how to implement a one-line for
loop to iterate through the following Python’s iterable objects:
myset1 = {"a", "b", "c", "d", "e", "f", "g"}
mydict1 = {"a": 1, "b": 2, "c": 3, "d": 4, "e": 5, "f": 6, "g": 7}
for x in myset1:
print(x)
for key, val in mydict1.items():
print(key, val)
Additionally, the range(start, stop, step)
function generates a sequence starting from the start
value and ending at the stop
value with a step size of step
.
Here’s an example using the range()
function with a one-line for
loop in Python:
for x in range(1, 5):
print(x)
List Comprehension in Python Using the One-Line for
Loop
List comprehension is a concise way to generate a new list from an existing one in Python. It allows you to apply operations to each element of the list and create a new list using a simple one-line for
loop.
Basic Syntax:
newlist = [expression for item in iterable]
Parameters:
newlist
: The name of the new list you want to create.expression
: The operation or transformation to apply to eachitem
.item
: The variable that represents each element in theiterable
.iterable
: The sequence or collection of items you want to iterate over.
Let’s explore an example of list comprehension using a one-line for
loop in Python. In this example, we’ll create a new list by squaring each element from an existing list:
mylist = [6, 2, 8, 3, 1]
newlist = [x**2 for x in mylist]
print(newlist)
Output:
[36, 4, 64, 9, 1]
In this code, we have a list called mylist
with values [6, 2, 8, 3, 1]
.
Next, we create a new list, newlist
, using a list comprehension. In this list comprehension, we iterate through each element x
in mylist
and calculate the square of each element by raising it to the power of 2
, denoted as x ** 2
.
The output of this code is the newly created newlist
, which contains the squares of the elements from mylist
. So, the output is [36, 4, 64, 9, 1]
.
List Comprehension in Python With the if ... else
Statement Using the One-Line for
Loop
List comprehension with an if ... else
statement is used to apply operations on some specific elements of the existing list to create a new list or filter elements from the existing list to create a new one.
Let’s see how to implement list comprehension with the if
and if...else
statements in Python using a one-line for
loop. In the following example, we add elements to a new list if they are odd numbers and discard them if they are even numbers:
mylist = [1, 4, 5, 8, 9, 11, 13, 12]
newlist = [x for x in mylist if x % 2 == 1]
print(newlist)
Output:
[1, 5, 9, 11, 13]
In this code, we start with a list named mylist
, which contains numbers: [1, 4, 5, 8, 9, 11, 13, 12]
.
Next, we create a new list, newlist
, using a list comprehension. In this comprehension, we iterate through each element x
in mylist
.
We include an if
condition to filter only the elements where x % 2 == 1
, which means we select only the odd numbers from mylist
.
The output of this code is the newlist
, which contains the odd numbers from the original list. So, the output is [1, 5, 9, 11, 13]
.
The following example utilizes a one-line if ... else
list comprehension to convert odd elements into even ones by adding 1
to them and leaves even elements unchanged, resulting in a new list of even numbers:
mylist = [1, 4, 5, 8, 9, 11, 13, 12]
newlist = [x + 1 if x % 2 == 1 else x for x in mylist]
print(newlist)
Output:
[2, 4, 6, 8, 10, 12, 14, 12]
In our code, we have a list called mylist
with these numbers: [1, 4, 5, 8, 9, 11, 13, 12]
.
We create a new list, newlist
, using a list comprehension. We iterate through each element x
in mylist
.
Then, we check if x
is an odd number (x % 2 == 1
). If it’s odd, we add 1
to it (x + 1
), and if it’s even, we leave it unchanged (x
).
The output of our code is the newlist
, which contains the elements from mylist
with odd numbers increased by 1
and even numbers left as they are. So, the output is [2, 4, 6, 8, 10, 12, 14, 12]
.
Conclusion
In this tutorial, we explored different methods for implementing one-line for
loops in Python. These one-liners can take the form of simple iterations through sequences or iterable objects, such as lists, arrays, sets, or dictionaries, as well as utilizing the range()
function for sequence generation.
We also delved into the power of list comprehensions, offering a concise way to create new lists by applying operations to existing elements. Moreover, we demonstrated the use of list comprehensions with if ... else
statements to filter and transform elements based on specific conditions.
These techniques empower Python programmers to efficiently manipulate data, perform operations on collections, and streamline their code for improved readability and functionality.