How to Fix the AttributeError: 'numpy.ndarray' Object Has No Attribute 'Append' in Python
-
the
AttributeError: 'numpy.ndarray' object has no attribute 'append'
in Python -
Fix the
AttributeError: 'numpy.ndarray' object has no attribute 'append'
in Python
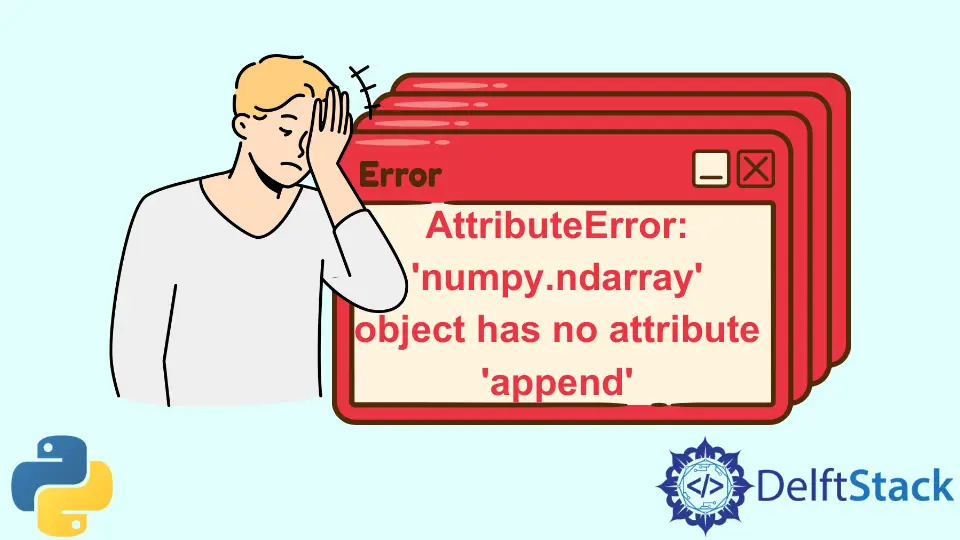
Like lists or arrays, NumPy doesn’t have the append()
method for the array; instead, we need to use the append()
method from NumPy. We can add multiple NumPy arrays using the append()
method.
the AttributeError: 'numpy.ndarray' object has no attribute 'append'
in Python
ndarray
is an n-dimensional NumPy array, which is useful for various purposes, like when we have multiple data types for a model. Here’s a simple example using this:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
print(f"Type: {type(arr)}")
print(f"Dimension: {arr.ndim}")
print(f"Shape: {arr.shape}")
print(f"Element data type: {arr.dtype}")
Output:
Type: <class 'numpy.ndarray'>
Dimension: 2
Shape: (2, 3)
Element data type: int32
Now, let’s try to append an array in the ndarray
object above. We will get the following error:
>>> arr.append([1,2])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'numpy.ndarray' object has no attribute 'append'
So, it is clear that the ndarray
type object doesn’t contain any method called append()
.
Fix the AttributeError: 'numpy.ndarray' object has no attribute 'append'
in Python
To append a new array in the ndarray
object, we need to ensure that the new array has the same dimension as the previous one inside the ndarray
.
Here’s how we will append the ndarray
:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
arr = np.append(arr, [[7, 8, 9]], axis=0)
print(arr)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Here, if you notice, we put the axis as 0
. Now, if we didn’t mention the axis, then this will happen:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
arr = np.append(arr, [[7, 8, 9]])
print(arr)
Output:
[1 2 3 4 5 6 7 8 9]
It just unwrapped all the elements and then made it one array!
Now, let’s observe what happens if we give an array that doesn’t have the same dimension:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
arr = np.append(arr, [[7, 8]], axis=0)
Output:
ValueError: all the input array dimensions for the concatenation axis must match exactly, but along dimension 1, the array at index 0 has size 3 and the array at index 1 has a size 2
Here we got the ValueError
for the dimension mismatch. To know more about the ndarray
of NumPy, visit this blog.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python