How to Count Number of Keys in Dictionary Python
-
Use the
len()
Function to Count the Number of Keys in a Python Dictionary - Create a User-Defined Function to Calculate the Number of Keys in a Python Dictionary
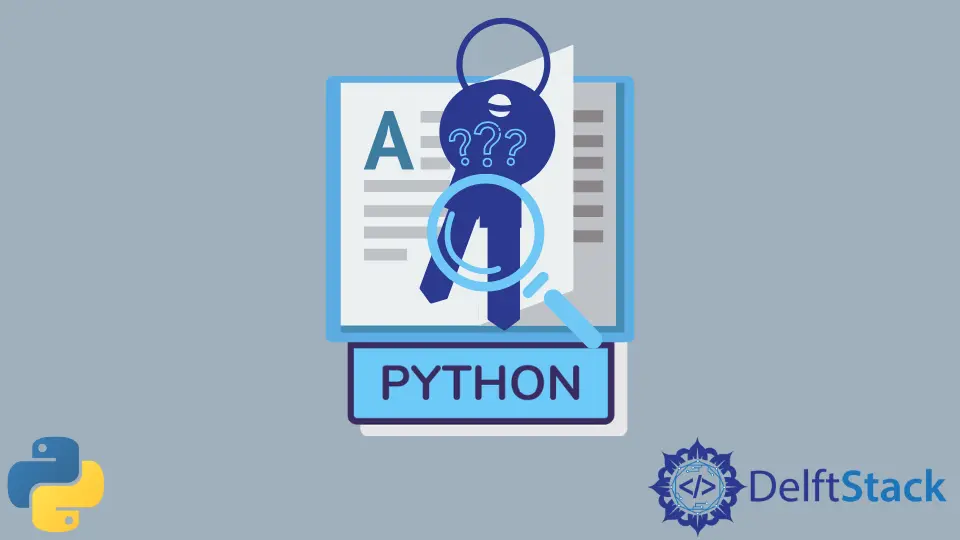
In this tutorial, we will discuss how to count the number of keys in a Python dictionary using the len()
function and also create our own function for the same purpose.
Use the len()
Function to Count the Number of Keys in a Python Dictionary
The len()
function in Python is used to return the total number of items present in an object. We can use the keys()
method of the dictionary to get a list of all the keys in the dictionary and count the total number using len()
.
dict1 = {"a": 1, "b": 2, "c": 3}
print(len(dict1.keys()))
Output:
3
We can also pass the dictionary directly to the len()
function, which returns the distinct total entries in the dictionary, which is the same as the number of the keys.
dict1 = {"a": 1, "b": 2, "c": 3}
print(len(dict1))
Output:
3
Create a User-Defined Function to Calculate the Number of Keys in a Python Dictionary
We can also create our own function to calculate the number of Python keys. We initialize a variable to 0, iterate over the dictionary using the enumerate()
, increment the variable in every iteration, and return it. The following code will explain this.
dict1 = {"a": 1, "b": 2, "c": 3}
def count_keys(dict):
count = 0
for i in enumerate(dict):
count += 1
return count
print(count_keys(dict1))
Output:
3
The enumerate()
function returns an enumerate object that attaches a counter variable to the dictionary’s keys and makes looping over the dictionary easily.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn