The nonlocal Keyword in Python
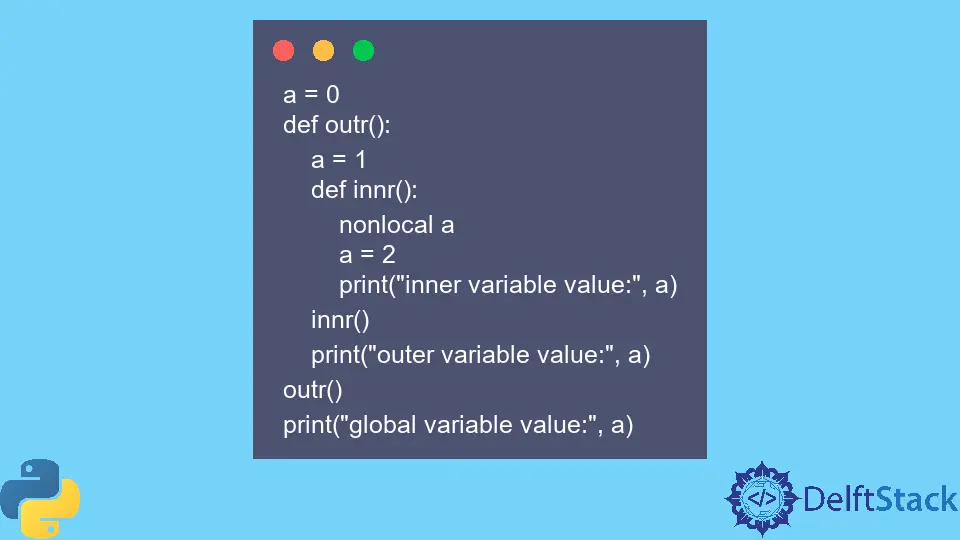
The nonlocal
keyword is utilized to set the scope of a particular variable. It is mostly used in cases where nesting occurs in a program.
In this tutorial, we will discuss the nonlocal
keyword in Python.
The nonlocal
keyword, when used, has a scope that is quite different from the general global
or local
variable. The nonlocal
keyword is utilized to work on variables under nested functions, in which case the scope of the given variable needs to be accessed in the outer function.
The nonlocal
function indicates that the given variable worked upon does not belong locally to the given nested function. However, this does not mean that the variable specified with the nonlocal
keyword is a global variable. Both nonlocal
and global
are different and work differently.
The following code uses the nonlocal
variable in Python.
a = 0
def outr():
a = 1
def innr():
nonlocal a
a = 2
print("inner variable value:", a)
innr()
print("outer variable value:", a)
outr()
print("global variable value:", a)
The above code provides the following output:
inner variable value: 2
outer variable value: 2
global variable value: 0
The above code explains how the nonlocal
keyword is utilized in Python. It provides a fascinating insight into how the nonlocal
keyword is different from the global
keyword.
The nonlocal
keyword rebinds the given variables outside the local scope but does not cover or rebind entirely to the global scope.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn