How to Fix the No Such File in Directory Error in Python
- Understanding the Error
- Method 1: Verify the File Path
- Method 2: Check File Permissions
- Method 3: Use Absolute Paths
- Method 4: Debugging with Print Statements
- Conclusion
- FAQ
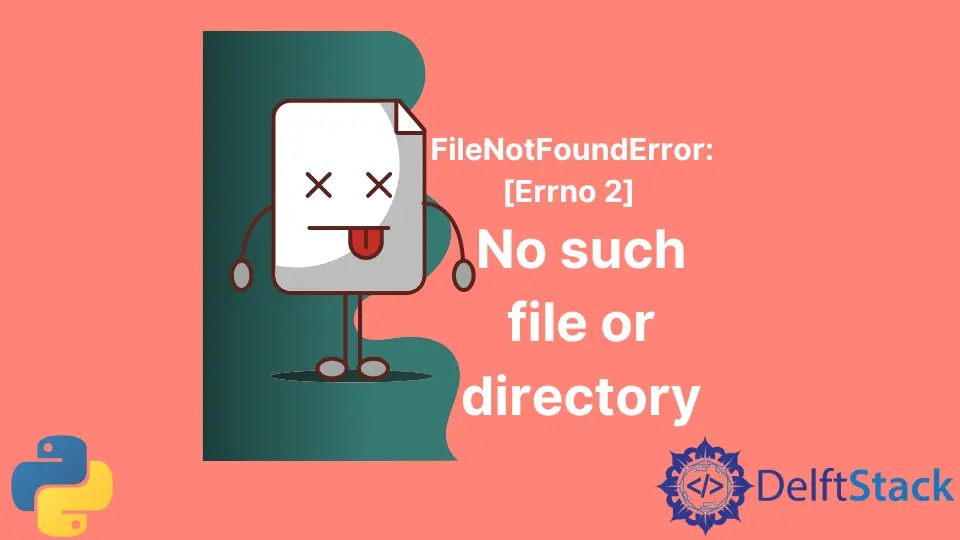
When working with Python, encountering the “No such file or directory” error can be frustrating. This common issue often arises when your code attempts to access a file that doesn’t exist in the specified path. Whether you’re reading a file, writing to it, or simply trying to list its contents, this error can halt your progress. But fear not!
In this article, we’ll explore practical solutions to resolve this error effectively. By the end, you’ll have a solid understanding of how to troubleshoot and fix this issue in your Python projects. Let’s dive in and get your code running smoothly again!
Understanding the Error
Before we jump into solutions, it’s essential to understand what causes the “No such file in directory” error. Essentially, Python raises this error when it cannot locate the file you’re trying to access. This can happen for several reasons:
- The file path is incorrect.
- The file doesn’t exist in the specified location.
- There are permission issues preventing access.
Understanding these factors is crucial for effectively resolving the error.
Method 1: Verify the File Path
One of the most common reasons for encountering this error is an incorrect file path. It’s essential to ensure that the path you are using in your code points to the correct file location. Here’s how you can check and fix the file path:
import os
file_path = "example.txt"
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
else:
print("File not found!")
Output:
File not found!
In this code snippet, we first import the os
module, which provides a way to interact with the operating system. We then define the file_path
variable with the name of the file we want to access. Using os.path.exists()
, we check if the file exists at the specified path. If it does, we proceed to open and read the file. If not, we print a message indicating that the file was not found. This method is straightforward and helps you quickly identify path issues.
Method 2: Check File Permissions
Sometimes, the error might not be due to the file’s existence but rather due to insufficient permissions to access it. If a file exists but your script cannot read it, you might encounter this error. Here’s how to check and adjust file permissions:
import os
file_path = "example.txt"
try:
with open(file_path, 'r') as file:
content = file.read()
print(content)
except PermissionError:
print("You do not have permission to access this file.")
except FileNotFoundError:
print("File not found!")
Output:
You do not have permission to access this file.
In this example, we attempt to open the file within a try
block. If the file exists but we lack the necessary permissions, Python raises a PermissionError
, which we catch and handle by printing an appropriate message. Additionally, we also handle the FileNotFoundError
to cover both scenarios. This method helps you determine if permissions are the issue.
Method 3: Use Absolute Paths
Using relative paths can sometimes lead to confusion, especially if your working directory changes. To avoid the “No such file in directory” error, consider using absolute paths. Here’s how you can implement this:
import os
file_path = os.path.abspath("example.txt")
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
else:
print("File not found!")
Output:
File not found!
In this code, we utilize the os.path.abspath()
function to convert the relative path to an absolute path. This ensures that Python looks for the file in the correct location, regardless of the current working directory. By checking if the file exists at this absolute path, we reduce the likelihood of encountering path-related errors. This method is particularly useful in larger projects where file structure can get complex.
Method 4: Debugging with Print Statements
If you’re still facing issues, adding print statements can help you debug the problem. By printing the current working directory and the file path, you can get insights into what might be going wrong. Here’s an example:
import os
file_path = "example.txt"
print("Current Working Directory:", os.getcwd())
print("File Path:", os.path.abspath(file_path))
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
else:
print("File not found!")
Output:
Current Working Directory: /path/to/your/directory
File Path: /path/to/your/directory/example.txt
File not found!
In this example, we print the current working directory using os.getcwd()
and the absolute file path. This additional information can help you verify if you’re looking in the right place for the file. By understanding the context in which your code runs, you can better diagnose the issue at hand.
Conclusion
Resolving the “No such file in directory” error in Python is often a matter of verifying your file paths, checking permissions, and using absolute paths. By following the methods outlined in this article, you can effectively troubleshoot and fix this common issue. Remember, understanding the underlying causes of the error is key to preventing it in the future. With these strategies at your disposal, you’ll be well-equipped to handle file-related challenges in your Python projects.
FAQ
-
What does the “No such file in directory” error mean?
This error indicates that Python cannot find the specified file at the given path. -
How can I check if a file exists in Python?
You can useos.path.exists(file_path)
to check if a file exists. -
What should I do if I get a permission error?
Ensure that you have the necessary permissions to access the file, or run your script with elevated privileges. -
How can I find the current working directory in Python?
Useos.getcwd()
to print the current working directory. -
Is it better to use relative paths or absolute paths in Python?
Absolute paths are generally more reliable, especially in larger projects, as they specify the exact location of the file.
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python