Nested List Comprehension in Python
- What is Nested List Comprehension?
- Example 3: Filtering with Nested List Comprehension
- Conclusion
- FAQ
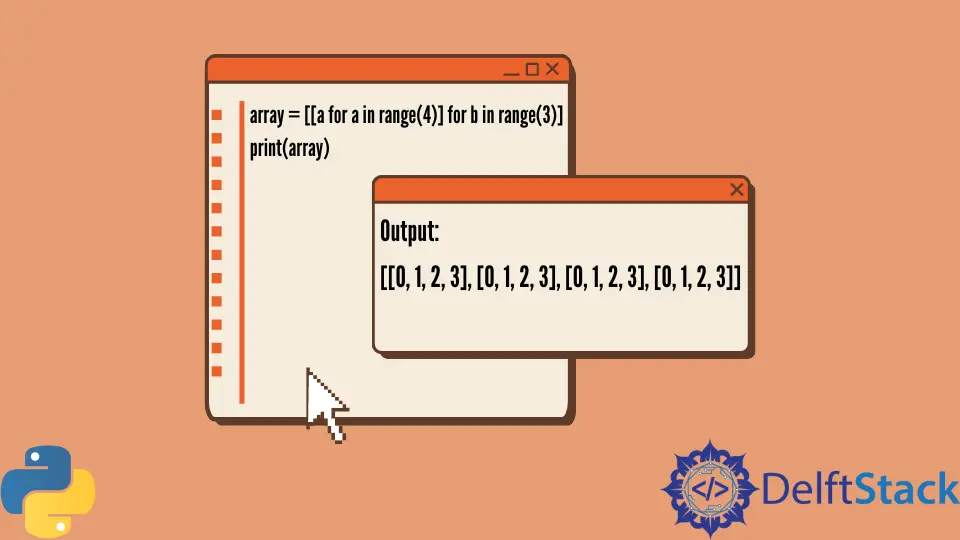
Nested list comprehension is a powerful feature in Python that allows you to create lists within lists in a concise and readable manner.
This tutorial will guide you through the fundamentals of nested list comprehension, showing you how to leverage this feature to simplify your code and enhance its efficiency. Whether you’re flattening a list of lists or creating complex data structures, understanding nested list comprehension will significantly improve your coding skills. By the end of this article, you’ll be equipped with practical examples and a clear understanding of how to use nested list comprehensions effectively in your Python projects.
What is Nested List Comprehension?
List comprehension is a compact way to process all or part of the elements in a sequence, such as a list or a string. When you nest one list comprehension inside another, you create a nested list comprehension. This allows you to iterate over multiple sequences and generate a new list based on the elements of these sequences.
For instance, suppose you have a matrix (a list of lists) and you want to extract specific elements or transform the entire structure. A nested list comprehension can help you achieve this in a single line of code, making your code cleaner and easier to read.
Example 1: Flattening a List of Lists
Let’s start with a straightforward example: flattening a list of lists. Imagine you have the following list:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [num for row in matrix for num in row]
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, the outer loop iterates over each row in the matrix, while the inner loop iterates over each number in that row. The result is a single flattened list containing all the numbers from the original matrix. This approach is not only concise but also enhances readability compared to traditional nested loops.
Example 2: Generating a Multiplication Table
Another practical application of nested list comprehension is generating a multiplication table. You can create a 2D list where each element represents the product of its row and column indices. Here’s how you can do that:
multiplication_table = [[i * j for j in range(1, 6)] for i in range(1, 6)]
Output:
[[1, 2, 3, 4, 5],
[2, 4, 6, 8, 10],
[3, 6, 9, 12, 15],
[4, 8, 12, 16, 20],
[5, 10, 15, 20, 25]]
In this example, the outer list comprehension iterates over the range from 1 to 5, representing the rows of the multiplication table. For each row, the inner list comprehension generates a list of products corresponding to each column. The result is a neatly structured multiplication table, demonstrating how nested list comprehension can simplify the creation of complex data structures.
Example 3: Filtering with Nested List Comprehension
Nested list comprehensions can also be used to filter elements based on certain conditions. Suppose you want to extract only the even numbers from a matrix. Here’s how you can do it:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
even_numbers = [num for row in matrix for num in row if num % 2 == 0]
Output:
[2, 4, 6, 8]
In this case, the inner loop iterates through each number in the row, while the if
statement filters out the odd numbers. The result is a list containing only the even numbers from the original matrix. This example showcases how nested list comprehensions can be combined with conditions to produce more refined outputs.
Conclusion
Nested list comprehension in Python is a versatile and efficient way to work with complex data structures. By mastering this technique, you can simplify your code, making it more readable and maintainable. Whether you are flattening lists, generating tables, or filtering data, nested list comprehension provides a powerful tool in your programming arsenal. As you continue to practice and implement this feature, you’ll find that it enhances your coding experience and efficiency.
FAQ
-
What is nested list comprehension in Python?
Nested list comprehension is a method to create lists within lists in a concise and readable manner. -
How does nested list comprehension improve code readability?
It allows developers to express complex operations in a single line, reducing the need for nested loops and enhancing clarity. -
Can you use conditions with nested list comprehension?
Yes, you can filter elements using conditions within the comprehension. -
What are some common use cases for nested list comprehension?
Common use cases include flattening lists, generating matrices, and filtering data. -
Is nested list comprehension limited to numerical data?
No, it can be used with any iterable data type, including strings and tuples.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python