Nested Dictionary in Python
- Creating a Nested Dictionary
- Accessing Values in a Nested Dictionary
- Modifying Values in a Nested Dictionary
- Adding New Entries to a Nested Dictionary
- Deleting Entries from a Nested Dictionary
- Conclusion
- FAQ
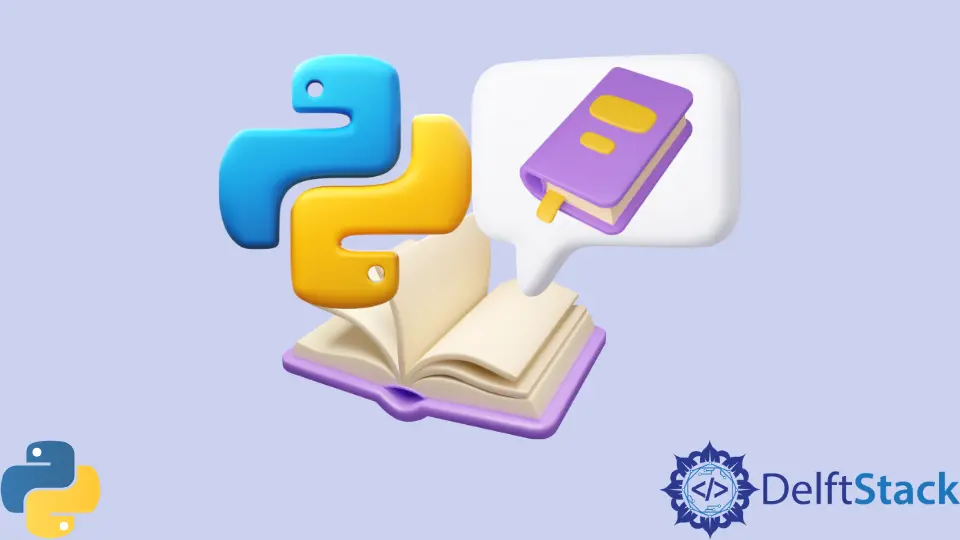
Nested dictionaries in Python are powerful data structures that allow you to store complex data in a hierarchical manner. They are essentially dictionaries within dictionaries, enabling you to organize data in a way that reflects real-world relationships. For instance, if you want to store information about students and their subjects, you can create a nested dictionary where each student is a key, and their subjects and grades are stored as another dictionary.
In this tutorial, we will explore how to create and use nested dictionaries in Python, complete with practical examples and explanations. Whether you’re a beginner or an experienced programmer, understanding nested dictionaries will enhance your coding skills and enable you to handle data more efficiently.
Creating a Nested Dictionary
Creating a nested dictionary in Python is straightforward. You can define a nested dictionary by simply including another dictionary as the value for a key in an existing dictionary. Here’s how you can do it:
students = {
"Alice": {
"Math": 85,
"Science": 90,
"English": 78
},
"Bob": {
"Math": 88,
"Science": 92,
"English": 80
}
}
In this example, the main dictionary students
has two keys: “Alice” and “Bob”. Each of these keys points to another dictionary that contains subjects and their corresponding grades. This structure allows you to easily access each student’s grades by referencing their name.
Output:
{
"Alice": {
"Math": 85,
"Science": 90,
"English": 78
},
"Bob": {
"Math": 88,
"Science": 92,
"English": 80
}
}
Creating a nested dictionary is a great way to group related data together. You can expand this structure further by adding more students or subjects as needed. The flexibility of nested dictionaries makes them suitable for various applications, from simple data storage to more complex data manipulation tasks.
Accessing Values in a Nested Dictionary
Once you have created a nested dictionary, accessing its values is simple. You can retrieve values by chaining keys together. For example, if you want to access Alice’s grade in Science, you can do it like this:
alice_science_grade = students["Alice"]["Science"]
print(alice_science_grade)
This code retrieves the value associated with the key “Science” from Alice’s dictionary. The output will display her grade in that subject.
Output:
90
Accessing values in a nested dictionary is efficient and intuitive. You can easily navigate through multiple layers of keys to get to the data you need. This feature is particularly useful when dealing with complex datasets where relationships between different data points need to be maintained.
Modifying Values in a Nested Dictionary
Modifying values in a nested dictionary is just as easy as accessing them. You can update a specific value by referencing the keys in the same way. For example, if you want to change Alice’s grade in Math, you can do it like this:
students["Alice"]["Math"] = 95
print(students["Alice"]["Math"])
This code updates Alice’s Math grade to 95 and then prints the updated value.
Output:
95
Modifying values in a nested dictionary allows you to keep your data current and accurate. Whether you are updating grades, adding new subjects, or even removing entries, the ability to easily change values makes nested dictionaries a versatile tool in Python programming.
Adding New Entries to a Nested Dictionary
You can also add new entries to a nested dictionary effortlessly. If you want to add a new student or a new subject for an existing student, you can do so by assigning a new key-value pair. Here’s how to add a new student named Charlie with his grades:
students["Charlie"] = {
"Math": 78,
"Science": 85,
"English": 88
}
print(students)
This code adds Charlie to the students
dictionary, along with his respective grades in different subjects.
Output:
{
"Alice": {
"Math": 95,
"Science": 90,
"English": 78
},
"Bob": {
"Math": 88,
"Science": 92,
"English": 80
},
"Charlie": {
"Math": 78,
"Science": 85,
"English": 88
}
}
Adding new entries is straightforward and allows your nested dictionary to grow as needed. This feature is particularly useful in applications where data is dynamic and subject to change over time.
Deleting Entries from a Nested Dictionary
Sometimes, you may need to remove an entry from a nested dictionary. Python provides a simple way to delete keys using the del
statement. If you want to remove Bob from the students
dictionary, you can do it like this:
del students["Bob"]
print(students)
This code deletes Bob’s entry from the students
dictionary.
Output:
{
"Alice": {
"Math": 95,
"Science": 90,
"English": 78
},
"Charlie": {
"Math": 78,
"Science": 85,
"English": 88
}
}
Deleting entries from a nested dictionary is straightforward and allows you to manage your data effectively. Whether you need to remove outdated information or simply want to clean up your dataset, this functionality makes it easy to maintain an organized structure.
Conclusion
In summary, nested dictionaries in Python are a powerful way to handle complex data structures. They allow you to store related information in a hierarchical manner, making it easy to access, modify, add, or delete entries. By understanding how to create and manipulate nested dictionaries, you can improve your data management skills and enhance your programming capabilities. As you continue to explore Python, you’ll find that nested dictionaries are invaluable for various applications, from data analysis to web development.
FAQ
-
What is a nested dictionary in Python?
A nested dictionary is a dictionary that contains another dictionary as its value, allowing for a hierarchical data structure. -
How do I access values in a nested dictionary?
You can access values by chaining keys together, likedictionary[key1][key2]
. -
Can I add new entries to a nested dictionary?
Yes, you can add new entries by assigning a new key-value pair to the nested dictionary.
-
How do I delete an entry from a nested dictionary?
You can delete an entry using thedel
statement followed by the key you want to remove. -
Are nested dictionaries mutable?
Yes, nested dictionaries are mutable, meaning you can change, add, or remove entries after they are created.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn