How to Fix NameError: The OS Module Is Not Defined in Python
- Understanding the NameError
- Solution 1: Importing the OS Module
- Solution 2: Checking for Typos
- Solution 3: Using Virtual Environments
- Conclusion
- FAQ
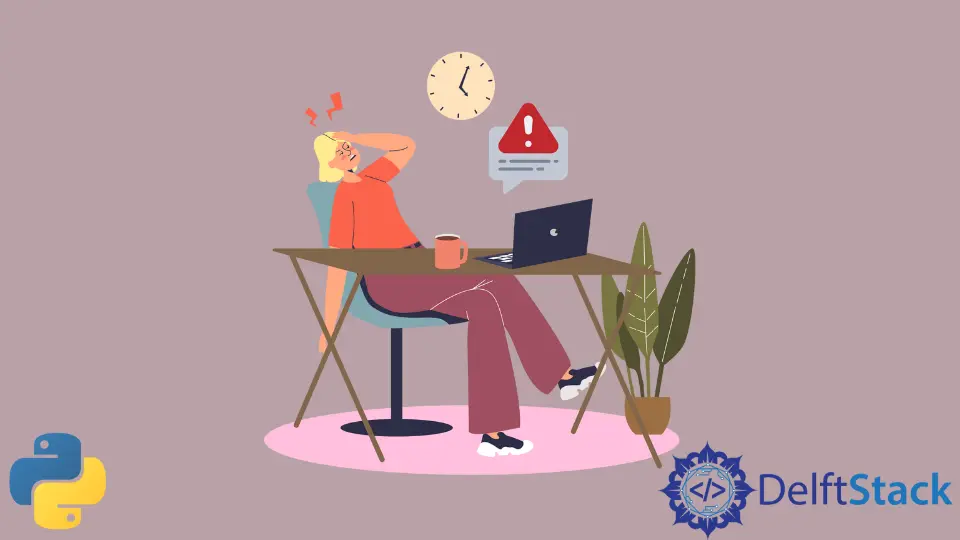
When working with Python, encountering errors can be frustrating, especially when they disrupt your workflow. One common issue developers face is the “NameError: the OS module is not defined.” This error typically arises when you attempt to use the OS module without importing it first.
In this tutorial, we will explore effective methods to resolve this error and ensure your Python scripts run smoothly. Whether you are a beginner or an experienced developer, understanding how to fix this issue will enhance your coding skills and improve your productivity. Let’s dive into the solutions and get you back on track!
Understanding the NameError
Before we jump into fixing the error, let’s clarify what it means. A NameError in Python occurs when the interpreter encounters a name that it does not recognize. In the case of the OS module, this usually means that you have not included the necessary import statement at the beginning of your script. The OS module provides a way to interact with the operating system, allowing you to perform tasks such as file manipulation, directory management, and environment variable access.
Solution 1: Importing the OS Module
The most straightforward way to resolve the NameError related to the OS module is to ensure that you have imported it correctly. To do this, simply add the import statement at the top of your Python script. Here’s how you can do it:
import os
current_directory = os.getcwd()
print("Current Directory:", current_directory)
Output:
Current Directory: /path/to/current/directory
In this example, we first import the OS module using the import os
statement. This line tells Python that we want to use the functionalities provided by the OS module. Next, we call the os.getcwd()
function, which returns the current working directory of the script. Finally, we print the current directory to the console. Without the import statement, Python would raise a NameError, indicating that the OS module is not defined.
Solution 2: Checking for Typos
Another common reason for encountering the NameError is typos in the module name. If you mistakenly type os
as Os
or OS
, Python will not recognize it. It’s crucial to ensure that you are using the correct casing when referencing module names. Here’s an example:
import os
file_list = os.listdir('.')
print("Files in Current Directory:", file_list)
Output:
Files in Current Directory: ['file1.txt', 'file2.py', 'subdirectory']
In this code snippet, we import the OS module and then use the os.listdir()
function to list all files in the current directory. If we had typed OS.listdir()
or Os.listdir()
, Python would have thrown a NameError because it is case-sensitive. Always double-check your module names to avoid this common pitfall.
Solution 3: Using Virtual Environments
Sometimes, the NameError can occur when you are working in a virtual environment and the OS module is not available. This can happen if the environment is not set up correctly. To fix this, you can create a new virtual environment and ensure that all necessary modules are installed. Here’s how to do it using Git commands:
-
Create a new virtual environment:
python -m venv myenv
-
Activate the virtual environment:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
- On Windows:
-
Install any required packages:
pip install -r requirements.txt
Output:
Successfully installed required packages
In this example, we create a new virtual environment named myenv
. After activating it, we can install necessary packages using the pip install
command. If your script relies on the OS module, make sure it is included in your requirements file or installed in the virtual environment. This approach can help you avoid conflicts and ensure that your environment is clean and functional.
Conclusion
Encountering the NameError: the OS module is not defined can be a common stumbling block for Python developers. However, by following the solutions outlined in this article—importing the OS module correctly, checking for typos, and using virtual environments—you can quickly resolve this issue. Remember, a little attention to detail goes a long way in programming. With these tips, you’ll be equipped to handle this error and continue your coding journey with confidence. Happy coding!
FAQ
- What causes the NameError: the OS module is not defined?
This error occurs when the OS module is not imported into your Python script.
-
How do I import the OS module in Python?
You can import the OS module by adding the lineimport os
at the beginning of your script. -
Can typos lead to a NameError?
Yes, typos in the module name, such as incorrect casing, can cause a NameError. -
What should I do if I encounter this error in a virtual environment?
Ensure your virtual environment is set up correctly and that all necessary packages are installed. -
How can I avoid NameErrors in my Python scripts?
Always double-check your imports and variable names for typos, and use virtual environments for project isolation.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python