How to Fix Name xrange Is Not Defined Error in Python
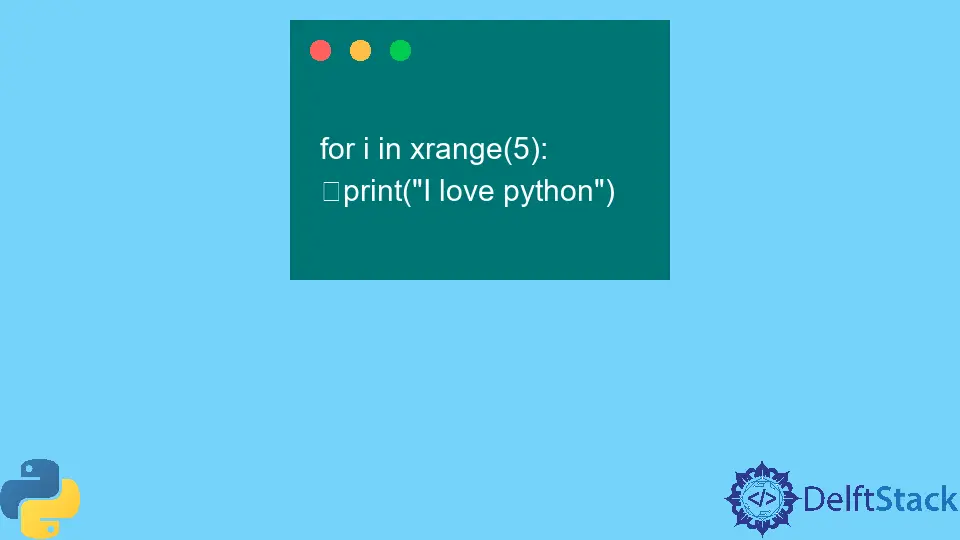
This tutorial will explore resolving the error name 'xrange' is not defined
in Python.
Resolve the name 'xrange' is not defined
Error in Python
Let us try to understand why this particular error occurs. Let us first start by trying to replicate this issue.
We can do this with the help of the following block of code.
for i in xrange(5):
print("I love python")
Note: It is important to install Python 3.0.0 or later on your device to replicate this error.
The code above results in the error below on your console.
line 1: name xrange is not defined
The main cause for this problem is that you have installed Python version 3.0.0 or later. The keyword xrange
does not work in any Python versions that occur after 2.9.0.
There are two ways to solve this problem.
-
Downgrading your Python version.
We can do this with the help of the following command.
conda install python=2.9.0
conda
environment only.-
The second method involves discarding the keyword
xrange
and replacing it withrange
. It does the same job thatxrange
used to do in the earlier versions.This can be understood better with the help of the following block of code.
for i in range(5): print("I love Python")
The output of the code above can be illustrated as follows.
I love Python
I love Python
I love Python
I love Python
I love Python
As we can see, the error has been resolved.
Thus, with the two techniques above, we can successfully resolve the error name 'xrange' is not defined
in Python versions 3.0.0 or later.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python