How to Fix Resolve the NameError: Global Name __File__ Is Not Defined Error in Python
Vaibhav Vaibhav
Feb 02, 2024
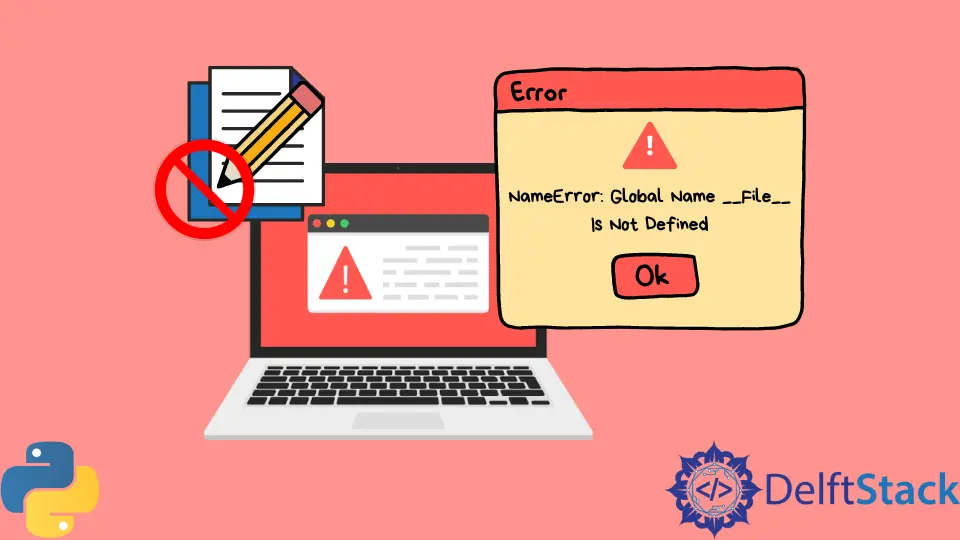
A dunder is a variable surrounded by double underscores. Python is a special variable meant for unique usage and stores special information.
The __file__
is a dunder in Python. It keeps the path to an imported Python module, and its value can be accessed as follows.
import math
import random
import numpy
print(random.__file__)
print(math.__file__)
print(numpy.__file__)
The Python interpreter raises the following error if this variable is not defined.
NameError: global name __file__ is not defined
This article will learn how to resolve this error in Python.
Resolve NameError: global name __file__ is not defined
Error in Python
This error arises when we try to access this variable inside the Python shell. All the code should be shifted to a Python file and executed using the following command from the terminal to fix this error.
python <file>.py <command line paramters>
Author: Vaibhav Vaibhav
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python