Mutex in Python
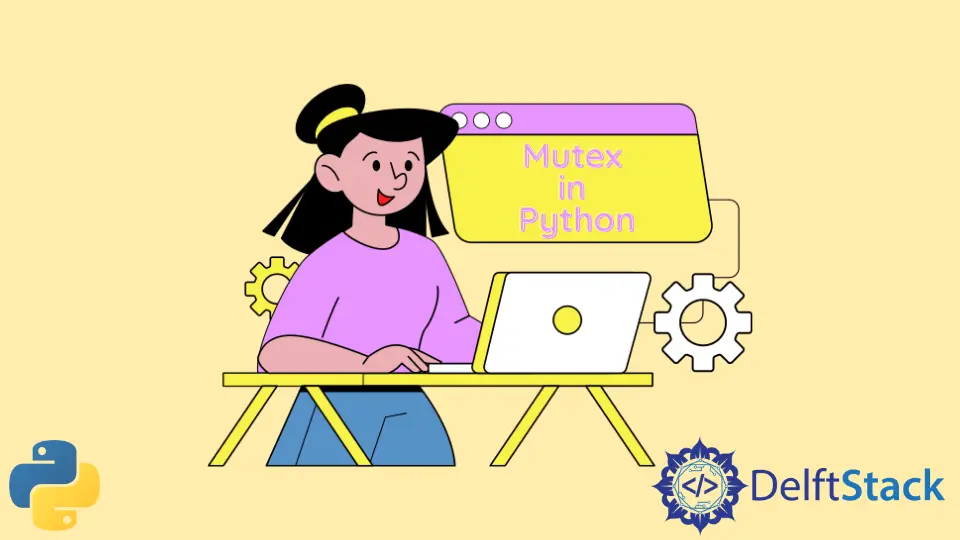
Mutex means Mutual Exclusion. It means that at a given specific time, only one thread can use a particular resource. If one program has multi-threads, then mutual exclusion restricts the threads to use that particular resource simultaneously. It locks the other threads and restricts their entry into the critical section.
This tutorial will demonstrate the use of mutex in Python.
To implement mutex in Python, we can use the lock()
function from the threading
module to lock the threads. If the second thread is about to finish before the first thread, it will wait for the first thread to finish. We lock the second thread to ensure this, and then we make it wait for the first thread to finish. And when the first thread is done, we release the lock of the second thread.
See the code given below.
import threading
import time
import random
mutex = threading.Lock()
class thread_one(threading.Thread):
def run(self):
global mutex
print("The first thread is now sleeping")
time.sleep(random.randint(1, 5))
print("First thread is finished")
mutex.release()
class thread_two(threading.Thread):
def run(self):
global mutex
print("The second thread is now sleeping")
time.sleep(random.randint(1, 5))
mutex.acquire()
print("Second thread is finished")
mutex.acquire()
t1 = thread_one()
t2 = thread_two()
t1.start()
t2.start()
Output:
The first thread is now sleeping
The second thread is now sleeping
First thread is finished
Second thread is finished
In this code, the second thread is not released until the first thread is done. The second thread waits for the first thread in the lock. The global
keyword is used in the code because both the thread uses it. Note that the print
statement comes right after the acquire
statement, not before, because as long as the thread is waiting, it has not finished yet.
Therefore, it is very important to lock the threads. Otherwise, it may crash the application if two threads share the same resource simultaneously.