The Modulo Operator (%) in Python
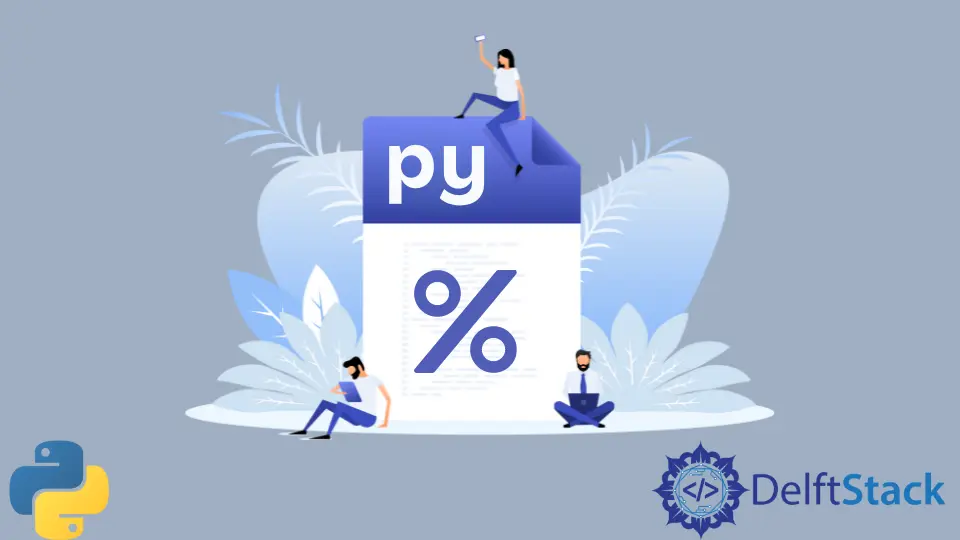
The symbol used to get the modulo in Python is the percentage mark %
.
This article will discuss and understand the meaning and use of the modulo operator(%) in Python.
Use the Modulo Operator in Arithmetic Operations
The modulo operator is used for arithmetic operations. Almost all languages require that this modulo operator has integer operands. However, Python Modulo is extremely versatile in this case.
Modulo is expressed as x%y
. An expression like x%y
corresponds to the remainder of x*y
. Its precedence is the same as the precedence of multiplication and division operators.
For example,
a = 10
b = 2
c = 11
print(a % b)
print(c % b)
Output:
0
1
Python’s modulo operation throws only one exception, the ZeroDivisionError
. This occurs when the modulo operator’s divider operand becomes zero. That means there can’t be a zero in the operand.
See the following code.
a = 5
b = 0
try:
print(a % b)
except ZeroDivisionError as err:
print("not divisible by zero!")
Output:
divisible by zero!
In the above example, we used the try...except
block to handle the exception. The %
raised the exception due to the value of variable b
being zero.
Use %
in String Operations in Python
In string formatting, %
is also used to replace values in a string by using values. We can use the %=
expression to replace values using a dictionary.
For example,
x = "abc_%(key)s"
x %= {"key": "def"}
print(x)
Output:
abc_def
We replaced the value of key
with value
using this operator. Its also used in string formatting when we wish to format the string based on a pattern. It acts as a placeholder for different values like strings, numbers, and more.
For example,
a = "abc"
b = 5
print("%s %d" % (a, b))
Output:
abc 5
For strings, we use %s
and for %d
for integers.