How to Check Python Module Version
-
Method 1: Using the
__version__
Attribute -
Method 2: Using the
pkg_resources
Module -
Method 3: Using the
pip
Command -
Method 4: Using the
importlib.metadata
Module - Conclusion
- FAQ
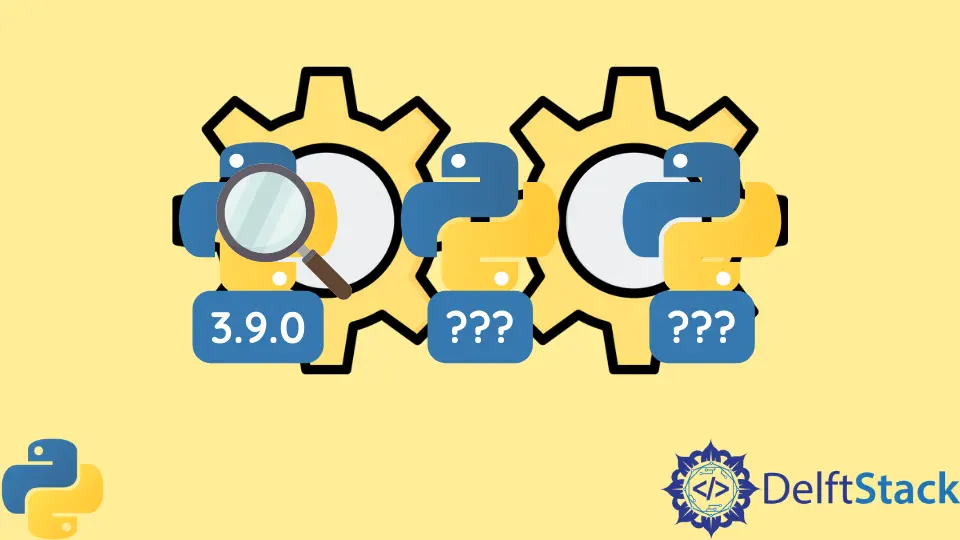
When working with Python, managing your modules and packages is crucial for maintaining a smooth development experience. One common task is checking the version of a specific module. Knowing the version can help you troubleshoot issues, ensure compatibility with other libraries, and take advantage of the latest features.
In this tutorial, we’ll explore various methods to find the version of a module installed in Python. Whether you’re a beginner or an experienced developer, these techniques will help you keep your Python environment organized and efficient.
Method 1: Using the __version__
Attribute
Many Python modules include a special attribute called __version__
that stores the version number as a string. This is one of the simplest ways to check the module version. Here’s how you can do it:
import numpy
print(numpy.__version__)
Output:
1.21.0
In this example, we import the NumPy library and print its version using the __version__
attribute. This method is straightforward and works for many popular libraries, including Pandas, Matplotlib, and Scikit-learn. However, not all modules define this attribute. If you try this method on a module that doesn’t have a __version__
, you’ll encounter an AttributeError
. Always check the documentation of the module for the best practices regarding version checking.
Method 2: Using the pkg_resources
Module
The pkg_resources
module, part of the setuptools
package, provides a robust way to retrieve package information, including the version. This method is particularly useful when dealing with packages that do not expose the __version__
attribute. Here’s how to use it:
import pkg_resources
version = pkg_resources.get_distribution("requests").version
print(version)
Output:
2.26.0
In this example, we use pkg_resources.get_distribution()
to access the version of the Requests library. This method is more versatile and works for any installed package, as long as it is properly registered with the package management system. The output will give you the exact version number, helping you verify compatibility with your project requirements. If the package is not found, it will raise a DistributionNotFound
exception, so you might want to handle that in your code.
Method 3: Using the pip
Command
Another effective way to check the version of installed Python modules is by using the pip
command directly from the terminal. This method is particularly handy when you want to get a quick overview of all installed packages and their versions. Here’s how to do it:
pip show numpy
Output:
Name: numpy
Version: 1.21.0
Summary: NumPy is the fundamental package for array computing with Python.
When you run this command, pip
displays detailed information about the specified package, including its version, summary, and location. This is a great way to get a comprehensive view of the package without writing any code. If you want to see all installed packages and their versions, simply run pip list
. This command will give you a list of all installed packages along with their respective versions, making it easy to manage your Python environment.
Method 4: Using the importlib.metadata
Module
Starting from Python 3.8, you can use the importlib.metadata
module to access package metadata, including version information. This method is particularly useful for applications that need to check module versions programmatically. Here’s how to use it:
from importlib.metadata import version
print(version("flask"))
Output:
2.0.1
In this example, we import the version
function from the importlib.metadata
module and use it to get the version of the Flask package. This method is elegant and leverages the built-in capabilities of Python, making it a great choice for modern applications. If the package is not installed, it will raise a PackageNotFoundError
, so be mindful of that when implementing this in your code. This approach is especially useful for applications that need to check dependencies at runtime.
Conclusion
Checking the version of a Python module is a fundamental skill for any developer. Whether you choose to use the __version__
attribute, the pkg_resources
module, the pip
command, or the importlib.metadata
module, each method offers unique advantages. By mastering these techniques, you can ensure that your projects run smoothly and remain compatible with various libraries. As you continue your journey in Python programming, keeping your modules updated and understanding their versions will greatly enhance your development efficiency.
FAQ
- How can I check the version of a module in Python?
You can check the version of a module using the__version__
attribute, thepkg_resources
module, thepip
command, or theimportlib.metadata
module.
-
What should I do if a module does not have a
__version__
attribute?
If a module does not have a__version__
attribute, you can use thepkg_resources
module or theimportlib.metadata
module to retrieve the version information. -
Can I check the version of multiple modules at once?
Yes, you can use thepip list
command to see the versions of all installed modules in your Python environment. -
How do I handle exceptions when checking module versions?
You can use try-except blocks to handle exceptions likeAttributeError
orDistributionNotFound
when checking module versions. -
Is there a difference between using
pip show
andpip list
?
Yes,pip show
provides detailed information about a specific package, whilepip list
displays a summary of all installed packages and their versions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn