How to Solve ModuleNotFoundError in Python
-
Use the Correct Module Name to Solve
ModuleNotFoundError
in Python -
Use the Correct Syntax to Solve
ModuleNotFoundError
in Python
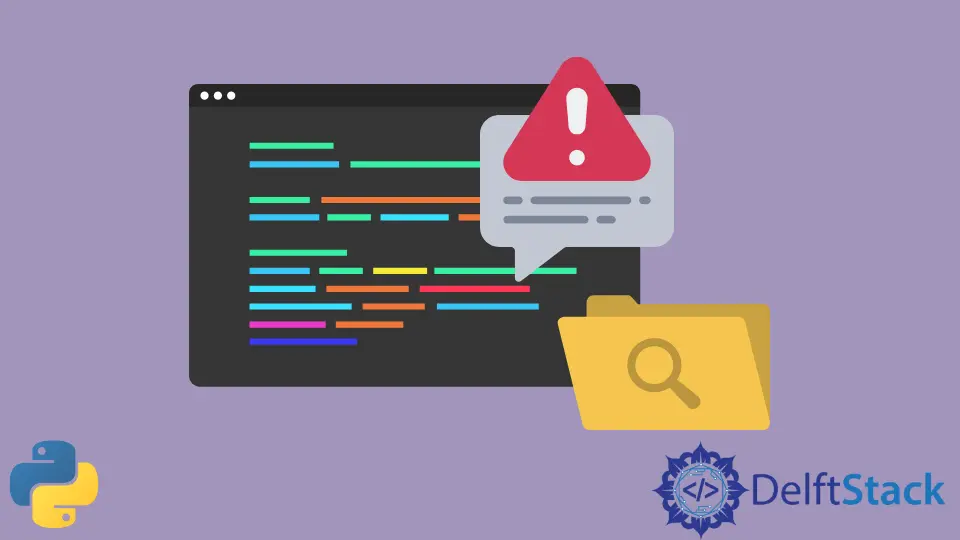
Modules are important for developing Python programs. With modules, we can separate different parts of a codebase for easier management.
When working with modules, understanding how they work and how we can import them into our code is important. Without this understanding or mistakes, we may experience different errors.
One example of such an error is ModuleNotFoundError
. In this article, we will discuss the way to resolve ModuleNotFoundError
within Python.
Use the Correct Module Name to Solve ModuleNotFoundError
in Python
Let’s create a simple Python codebase with two files, index.py
and file.py
, where we import file.py
into the index.py
file. Both files are within the same directory.
The file.py
file contains the code below.
class Student:
def __init__(self, firstName, lastName):
self.firstName = firstName
self.lastName = lastName
The index.py
file contains the code below.
import fiIe
studentOne = fiIe.Student("Isaac", "Asimov")
print(studentOne.lastName)
Now, let’s run index.py
. The output of our code execution is below.
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\index.py", line 1, in <module>
import fiIe
ModuleNotFoundError: No module named 'fiIe'
We have a ModuleNotFoundError
. If you look closely, you will notice that the import statement has a typographical error where file
is written as fiIe
with the l
replaced with a capital I
.
Therefore, if we use the wrong name, a ModuleNotFoundError
can be thrown at us. Be careful when writing your module names.
Now, let’s correct it and get our code running.
import file
studentOne = file.Student("Isaac", "Asimov")
print(studentOne.lastName)
The output of the code:
Asimov
Also, we could rewrite the import
statement using the from
keyword and import
just the Student
class. This is useful for cases where we don’t want to import all the functions, classes, and methods present within the module.
from file import Student
studentOne = Student("Isaac", "Asimov")
print(studentOne.lastName)
We will get the same output as the last time.
Use the Correct Syntax to Solve ModuleNotFoundError
in Python
We can get a ModuleNotFoundError
when we use the wrong syntax when importing another module, especially when working with modules in a separate directory.
Let’s create a more complex codebase using the same code as the last section but with some extensions. To create this codebase, we need the project structure below.
Project/
data/
file.py
welcome.py
index.py
With this structure, we have a data
package that houses the file
and welcome
modules.
In the file.py
file, we have the code below.
class Student:
def __init__(self, firstName, lastName):
self.firstName = firstName
self.lastName = lastName
In the welcome.py
, we have the code below.
def printWelcome(arg):
return "Welcome to " + arg
The index.py
contains code that tries to import file
and welcome
and uses the class Student
and function printWelcome
.
import data.welcome.printWelcome
import data.file.Student
welcome = printWelcome("Lagos")
studentOne = Student("Isaac", "Asimov")
print(welcome)
print(studentOne.firstName)
The output of running index.py
:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\index.py", line 1, in <module>
import data.welcome.printWelcome
ModuleNotFoundError: No module named 'data.welcome.printWelcome'; 'data.welcome' is not a package
The code tried to import the function printWelcome
and class Student
using the dot operator directly instead of using the from
keyword or the __init__.py
for easy binding for submodules. By doing this, we have a ModuleNotFoundError
thrown at us.
Let’s use the correct import
statement syntax to prevent ModuleNotFoundError
and import the function and class directly.
from data.file import Student
from data.welcome import printWelcome
welcome = printWelcome("Lagos")
studentOne = Student("Isaac", "Asimov")
print(welcome)
print(studentOne.firstName)
The output of the code:
Welcome to Lagos
Isaac
We can bind the modules (file
and welcome
) within the data
package to its parent namespace. To do this, we need the __init__.py
file.
In the __init__.py
file, we import all the modules and their functions, classes, or objects within the package to allow easy management.
from .file import Student
from .welcome import printWelcome
Now, we can write our index.py
more succinctly and with a good binding to the parent namespace, data
.
from data import Student, printWelcome
welcome = printWelcome("Lagos")
studentOne = Student("Isaac", "Asimov")
print(welcome)
print(studentOne.firstName)
The output will be the same as the last code execution.
To prevent the ModuleNotFoundError
error message, ensure you don’t have a wrong import
statement or typographical errors.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python ModuleNotFoundError
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python