Lookup Table in Python
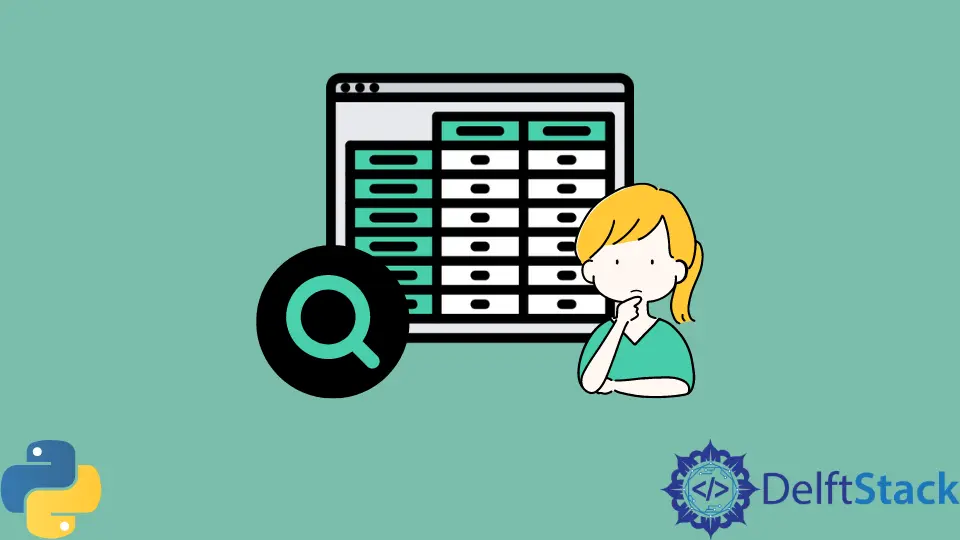
Lookup Table is used to access the values of the database from tables easily. Using this, we can quickly get the output values of corresponding input values from the given table. In python, lookup tables are also known as dictionaries.
This tutorial will demonstrate how to use a lookup table in Python.
Let’s make a dictionary that stores the key-value pairs. The keys are given numerical values, and the values of keys are assigned the string representation. Now, to get the value, we will use the key using the lookup table operation.
See the code given below.
dictionary = {18: "Eighteen", 11: "Eleven", 24: "Twenty-Four", 30: "Thirty"}
print(dictionary[11])
Output:
Eleven
Here, we have chosen the key as 11
. Therefore, we got the corresponding value of 11
as output. Note the 11
here is not the index but the key whose value we are looking for.
Dictionaries are not restricted to integers value only.
For Example,
myCars = {"audi": 99, "jaguar": 32, "mercedes": 59}
print(myCars["audi"])
Output:
99