Locust in Python
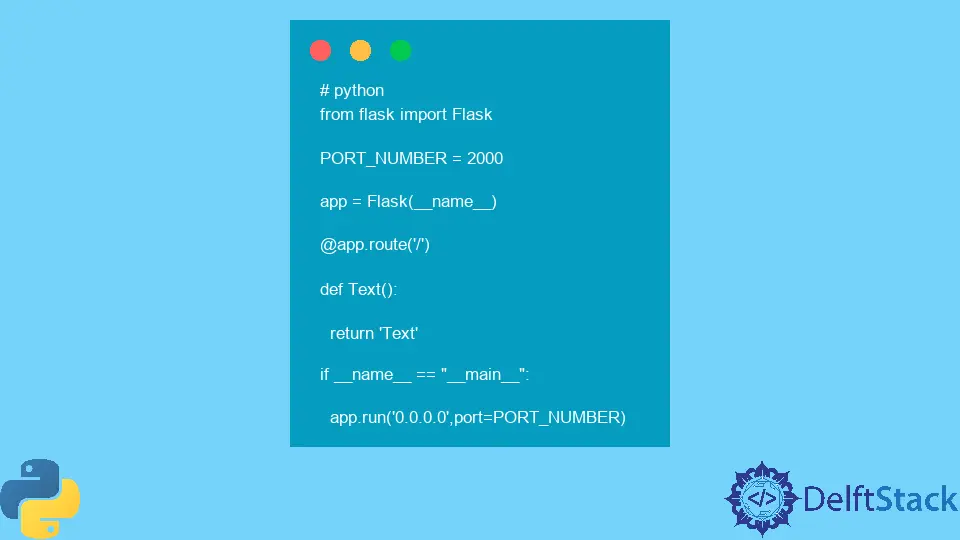
Load testing is an essential part of ensuring that applications can handle user traffic efficiently. When it comes to Python, Locust is a powerful tool that simplifies this process.
In this tutorial, we will explore what Locust is, how to set it up, and how to use it effectively for load testing your applications. Whether you’re a developer looking to optimize your web application or a QA engineer aiming to ensure performance under stress, this guide will provide you with the insights you need. We will also include practical code examples to help you get started with Locust in Python.
What is Locust?
Locust is an open-source load testing tool written in Python. It allows you to define user behavior in code, simulating multiple users accessing your application simultaneously. This is particularly useful for identifying performance bottlenecks and ensuring that your application can handle high traffic volumes. Locust’s web-based user interface makes it easy to monitor test results in real-time, providing valuable insights into how your application performs under load.
Setting Up Locust
Before you can start using Locust, you need to install it. The easiest way to do this is via pip, Python’s package manager. In your terminal, run the following command:
pip install locust
Once installed, you can verify the installation by checking the version:
locust --version
Output:
locust 2.x.x
After confirming the installation, you can create a new Python file for your load testing script. For example, create a file named locustfile.py
. This is where you’ll define the user behavior you want to simulate.
In locustfile.py
, you can start by importing the necessary modules:
from locust import HttpUser, task, between
class UserBehavior(HttpUser):
wait_time = between(1, 5)
@task
def load_test(self):
self.client.get("/")
In this code snippet, we define a user class that extends HttpUser
. The wait_time
attribute simulates the time a user waits between tasks, while the load_test
method makes a GET request to the root URL of your application.
Running Locust
To run your Locust test, navigate to the directory where your locustfile.py
is located and execute the following command:
locust -f locustfile.py --host http://your-application-url.com
Output:
Starting web interface at http://0.0.0.0:8089
After running this command, open your web browser and go to http://localhost:8089
. You will see the Locust web interface, where you can specify the number of users to simulate and the spawn rate. Once you start the test, Locust will begin sending requests to your application according to the defined behavior.
In the web interface, you can monitor various metrics such as response times, request failures, and the number of requests per second. This real-time data is invaluable for identifying performance issues and understanding how your application behaves under load.
Analyzing Results
After running your load test, it’s crucial to analyze the results to gain insights into your application’s performance. Locust provides several metrics, including:
- Response times: The time taken for your application to respond to requests.
- Requests per second: The number of requests your application can handle per second.
- Failure rates: The percentage of requests that resulted in errors.
These metrics help you pinpoint areas that need improvement. For instance, if you notice high response times or failure rates, it may indicate that your application is struggling to handle the load. You can then investigate further by looking into server logs, database performance, or optimizing your code.
Conclusion
Locust is a powerful tool for load testing in Python, providing a flexible and user-friendly way to simulate user behavior and analyze application performance. By following the steps outlined in this tutorial, you’ll be well-equipped to set up and run load tests on your applications. Remember that regular load testing is essential for maintaining optimal performance and ensuring a seamless user experience. With Locust, you can confidently identify and address potential bottlenecks before they impact your users.
FAQ
-
What is Locust used for?
Locust is used for load testing web applications to ensure they can handle high traffic volumes. -
Is Locust easy to set up?
Yes, Locust is easy to set up using pip, and you can quickly create load tests by defining user behavior in Python.
-
Can I monitor results in real-time with Locust?
Yes, Locust provides a web-based interface that allows you to monitor test results in real-time. -
Does Locust support distributed load testing?
Yes, Locust supports distributed load testing, allowing you to run tests across multiple machines. -
What programming language is Locust written in?
Locust is written in Python, making it accessible for developers familiar with the language.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn