How to Convert List to Tuple in Python
- Method 1: Using the tuple() Function
- Method 2: Using List Comprehension
- Method 3: Using the * Operator for Unpacking
-
Method 4: Using a
for
Loop - Conclusion
- FAQ
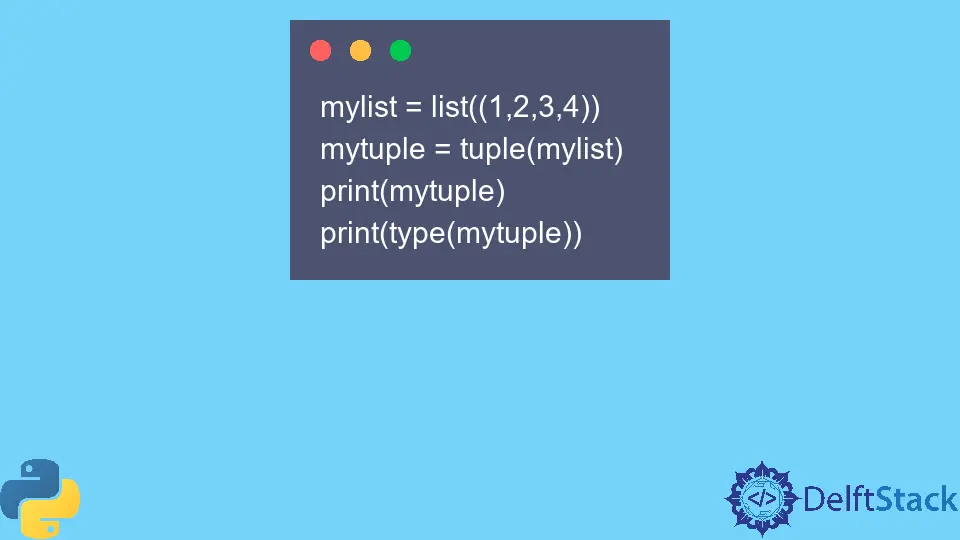
Converting a list to a tuple in Python is a common task that every programmer encounters. Whether you’re managing data structures or optimizing performance, understanding how to effectively make this conversion can enhance your coding efficiency.
In this tutorial, we will explore various methods to convert a list to a tuple, each with clear examples and explanations. By the end of this article, you will have a solid understanding of how to perform this conversion in Python, along with practical code snippets that you can implement in your own projects. Let’s dive in and learn how to convert lists to tuples effortlessly!
Method 1: Using the tuple() Function
The simplest and most common method to convert a list to a tuple in Python is by using the built-in tuple()
function. This function takes an iterable, such as a list, and converts it into a tuple. Here’s how it works:
my_list = [1, 2, 3, 4, 5]
my_tuple = tuple(my_list)
print(my_tuple)
Output:
(1, 2, 3, 4, 5)
In this example, we first define a list called my_list
containing integers from 1 to 5. We then pass this list to the tuple()
function, which converts it into a tuple and stores it in my_tuple
. Finally, we print the resulting tuple. This method is efficient and straightforward, making it ideal for quick conversions. The tuple()
function works with any iterable, so you can use it with other data structures as well, such as strings or sets, making it a versatile tool in your Python toolkit.
Method 2: Using List Comprehension
Another method to convert a list to a tuple is by using list comprehension along with the tuple()
function. This approach can be particularly useful if you want to apply some transformation or condition while converting. Here’s an example:
my_list = [1, 2, 3, 4, 5]
my_tuple = tuple(x for x in my_list)
print(my_tuple)
Output:
(1, 2, 3, 4, 5)
In this example, we utilize a generator expression within the tuple()
function. The generator expression iterates over each element x
in my_list
, and the tuple()
function collects these elements into a tuple. This method allows for more flexibility, as you can easily modify the generator expression to include conditions or transformations, such as filtering out certain values or applying a mathematical operation. For instance, if you only wanted even numbers, you could modify the expression to x for x in my_list if x % 2 == 0
. This versatility makes list comprehension a powerful method for tuple conversion.
Method 3: Using the * Operator for Unpacking
A less common but interesting method to convert a list to a tuple is by using the unpacking operator *
. This operator allows you to unpack the elements of the list directly into a tuple. Here’s how it works:
my_list = [1, 2, 3, 4, 5]
my_tuple = (*my_list,)
print(my_tuple)
Output:
(1, 2, 3, 4, 5)
In this example, we define a list my_list
and then create a tuple my_tuple
using the unpacking operator. By placing the asterisk *
before my_list
, we effectively unpack all the elements of the list into the tuple. The trailing comma is necessary to indicate that we are creating a tuple, as otherwise, Python would interpret it as a simple parentheses expression. This method is particularly useful when you want to combine multiple lists or other iterables into a single tuple. It’s a neat trick that can simplify your code in certain scenarios.
Method 4: Using a for
Loop
If you prefer a more manual approach, you can also convert a list to a tuple using a for loop. This method is straightforward and allows you to see the conversion process step by step. Here’s an example:
my_list = [1, 2, 3, 4, 5]
my_tuple = ()
for item in my_list:
my_tuple += (item,)
print(my_tuple)
Output:
(1, 2, 3, 4, 5)
In this example, we start with an empty tuple called my_tuple
. We then iterate over each item
in my_list
and add it to my_tuple
using the +=
operator. This effectively builds the tuple one element at a time. While this method works, it is less efficient than the previous methods, particularly for large lists, as tuples are immutable. Each concatenation creates a new tuple, which can lead to increased memory usage and slower performance. Nevertheless, this method can be useful for educational purposes or when you want to implement additional logic during the conversion process.
Conclusion
In this tutorial, we explored various methods to convert a list to a tuple in Python, including using the built-in tuple()
function, list comprehension, the unpacking operator, and a for loop. Each method has its unique advantages and use cases, allowing you to choose the one that best fits your needs. Whether you’re looking for simplicity, flexibility, or a more manual approach, Python provides you with the tools to efficiently convert lists to tuples. Understanding these methods will enhance your programming skills and help you write more effective Python code. Happy coding!
FAQ
- What is the difference between a list and a tuple in Python?
A list is mutable, meaning you can change its contents, while a tuple is immutable, meaning once created, it cannot be modified. - Can I convert a nested list to a tuple?
Yes, you can convert a nested list to a tuple using the same methods. However, the inner lists will also be converted to tuples. - Is it possible to convert a tuple back to a list?
Yes, you can convert a tuple back to a list using the built-inlist()
function. - What are the performance implications of using tuples over lists?
Tuples are generally faster than lists for iteration and are more memory efficient due to their immutability.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python