How to Create List of Zeros in Python
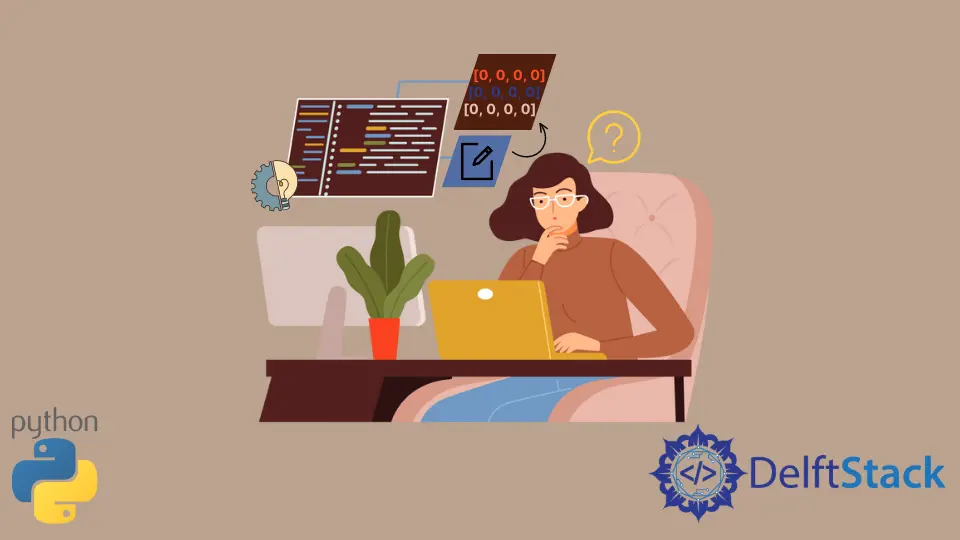
Creating a list of zeros in Python is a common task that can be useful in various programming scenarios, such as initializing arrays for mathematical computations or preparing data structures for algorithms. Whether you’re a beginner or an experienced programmer, knowing how to efficiently generate a list filled with zeros can streamline your coding process.
In this tutorial, we will explore several methods to create a list of zeros in Python, complete with code examples and detailed explanations. By the end of this article, you will have a solid understanding of how to tackle this task effectively.
Using List Comprehension
One of the most Pythonic ways to create a list of zeros is by using list comprehension. This method is concise and easy to read, making it a popular choice among developers.
zeros_list = [0 for _ in range(10)]
Output:
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In this example, we create a list called zeros_list
that contains ten zeros. The expression inside the brackets [0 for _ in range(10)]
generates a zero for each iteration of the loop that runs ten times. The underscore _
is used as a throwaway variable since we don’t need its value. This method is not only efficient but also very readable, making it a great choice for anyone looking to generate a list of zeros quickly.
Using the *
Operator
Another straightforward method for creating a list of zeros involves using the multiplication operator (*
). This approach is both efficient and clean, allowing you to specify the number of zeros you want in a single line.
zeros_list = [0] * 10
Output:
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
Here, we multiply a list containing a single zero [0]
by 10
, resulting in a new list that contains ten zeros. This method is particularly useful when you need a quick and easy way to initialize a list without the overhead of a loop. It’s a favorite among Python developers for its simplicity and efficiency.
Using the numpy
Library
If you are working with numerical data, the numpy
library provides a powerful tool for creating arrays filled with zeros. This method is especially beneficial for larger datasets or when you need to perform mathematical operations.
import numpy as np
zeros_array = np.zeros(10)
Output:
[0. 0. 0. 0. 0. 0. 0. 0. 0. 0.]
In this example, we first import the numpy
library and then use the np.zeros()
function to create an array of zeros. The argument 10
specifies the size of the array. The output is a NumPy array, which is slightly different from a standard Python list, as it is designed for numerical computations and offers various functionalities. This method is ideal for data science and engineering applications where performance is key.
Using a Loop
While using a loop may not be the most efficient method compared to the previous approaches, it is still a valid technique for creating a list of zeros. This method can be useful if you need to perform additional operations during the list creation process.
zeros_list = []
for _ in range(10):
zeros_list.append(0)
Output:
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
In this example, we initialize an empty list called zeros_list
. We then use a for
loop to append a zero to the list for each iteration, resulting in a list of ten zeros. While this method is straightforward, it is generally less efficient than list comprehension or the *
operator, particularly for larger lists. Nonetheless, it provides flexibility for more complex scenarios where additional logic might be needed during the list creation.
Conclusion
Creating a list of zeros in Python is a simple yet essential skill for any programmer. Whether you choose list comprehension, the multiplication operator, the numpy
library, or a loop, each method has its advantages and can be applied based on your specific needs. As you become more familiar with these techniques, you’ll find that they can significantly enhance your coding efficiency and effectiveness. Now that you have a variety of methods at your disposal, you can tackle any project that requires initializing lists with zeros.
FAQ
-
What is the most efficient way to create a list of zeros in Python?
The most efficient ways are using list comprehension or the multiplication operator. -
Can I create a list of zeros with a different data type?
Yes, you can create a list of any type by replacing0
with the desired value. -
Is using numpy the best option for small lists?
For small lists, it’s generally better to use list comprehension or the multiplication operator, as numpy is more suited for larger datasets.
-
How do I create a multi-dimensional array of zeros?
You can use numpy’snp.zeros((rows, columns))
to create a multi-dimensional array. -
Can I modify the values in a list created with these methods?
Yes, once created, you can modify the values in the list as needed.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python