How to Create List of Lists in Python
-
Use the
append()
Function to Create a List of Lists in Python - Use the List Comprehension Method to Create a List of Lists in Python
-
Use the
for
Loop to Create a List of Lists in Python
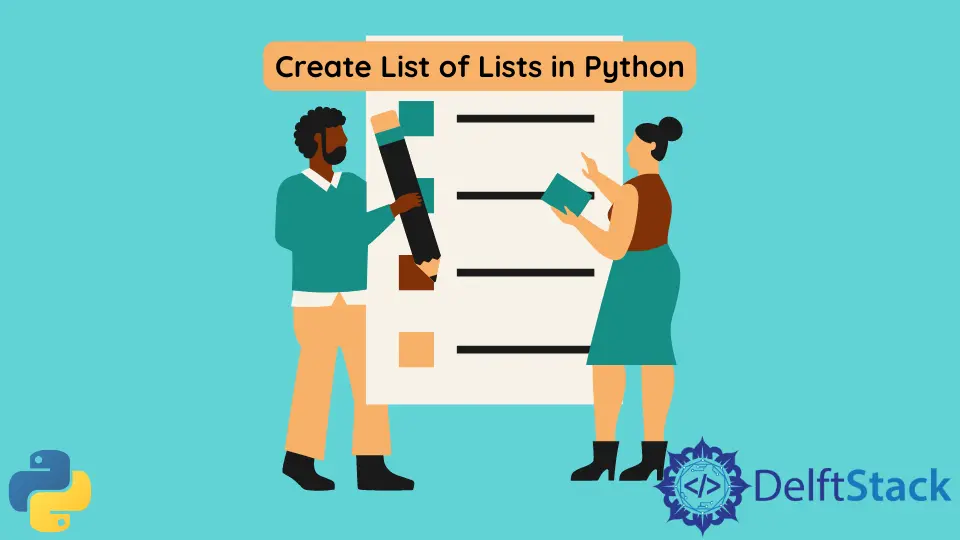
We can have a list of many types in Python, like strings, numbers, and more. Python also allows us to have a list within a list called a nested list or a two-dimensional list.
In this tutorial, we will learn how to create such lists.
Use the append()
Function to Create a List of Lists in Python
We can add different lists to a common list using the append()
function. It adds the list as an element to the end of the list.
The following code will explain this.
l1 = [1, 2, 3]
l2 = [4, 5, 6]
l3 = [7, 8, 9]
lst = []
lst.append(l1)
lst.append(l2)
lst.append(l3)
print(lst)
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Note that such a two-dimensional list that contains integer or float values can be considered as a matrix.
Use the List Comprehension Method to Create a List of Lists in Python
List comprehension is a straightforward yet elegant way to create lists in Python. We use the for
loops and conditional statements within the square brackets to create lists using this method.
We can create nested lists using this method, as shown below.
l1 = [1, 2, 3]
lst = [l1 for i in range(3)]
lst
Output:
[[1, 2, 3], [1, 2, 3], [1, 2, 3]]
Use the for
Loop to Create a List of Lists in Python
We can create a more complex list of lists by explicitly using the append()
function with the for
loop. We will use nested loops in this method. For example,
lst = []
for i in range(3):
lst.append([])
for j in range(3):
lst[i].append(j)
print(lst)
Output:
[[0, 1, 2], [0, 1, 2], [0, 1, 2]]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python