Linear Search in Python
Harshit Jindal
Oct 10, 2023
Python
Python Algorithm
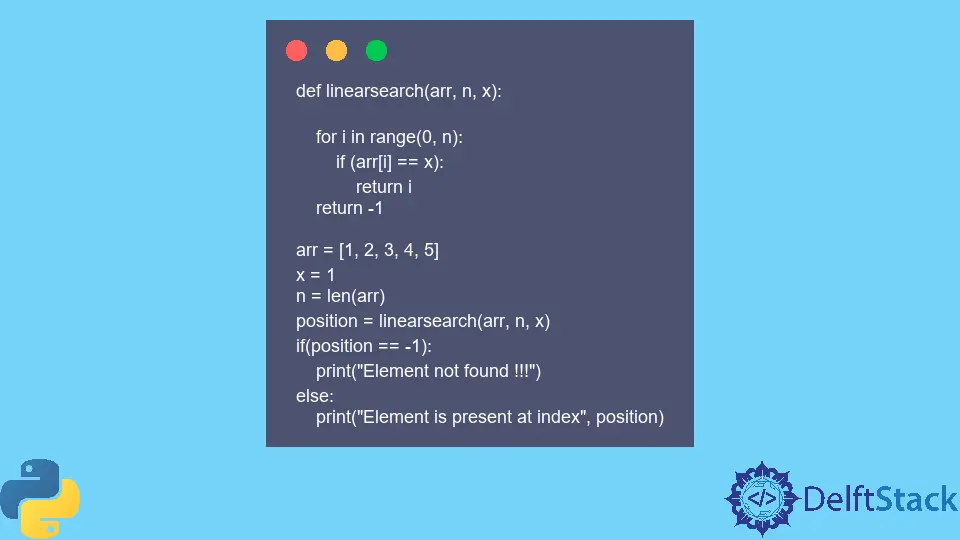
Note
If you want to understand Linear Search in detail then refer to the Linear Search Algorithm article.
Linear Search Algorithm
Let us assume that we have an unsorted array A[]
containing n
elements, and we want to find an element - X
.
-
Traverse all elements inside the array starting from the leftmost element using a
for
loop and do the following:- If the value of
A[i]
matches withX
, then return the indexi
. (If there can be multiple elements matchingX
, then instead of returning the indexi
, either print all indexes or store all the indexes in an array and return that array.) - Else move on to the next element.
- If it is at the last element of the array, quit the
for
loop.
- If the value of
-
If none of the elements matched, then return
-1
.
Linear Search Python Implementation
def linearsearch(arr, n, x):
for i in range(0, n):
if arr[i] == x:
return i
return -1
arr = [1, 2, 3, 4, 5]
x = 1
n = len(arr)
position = linearsearch(arr, n, x)
if position == -1:
print("Element not found !!!")
else:
print("Element is present at index", position)
Output:
Element is found at index: 1
The time complexity of the above algorithm is O(n)
.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn