Line Continuation in Python
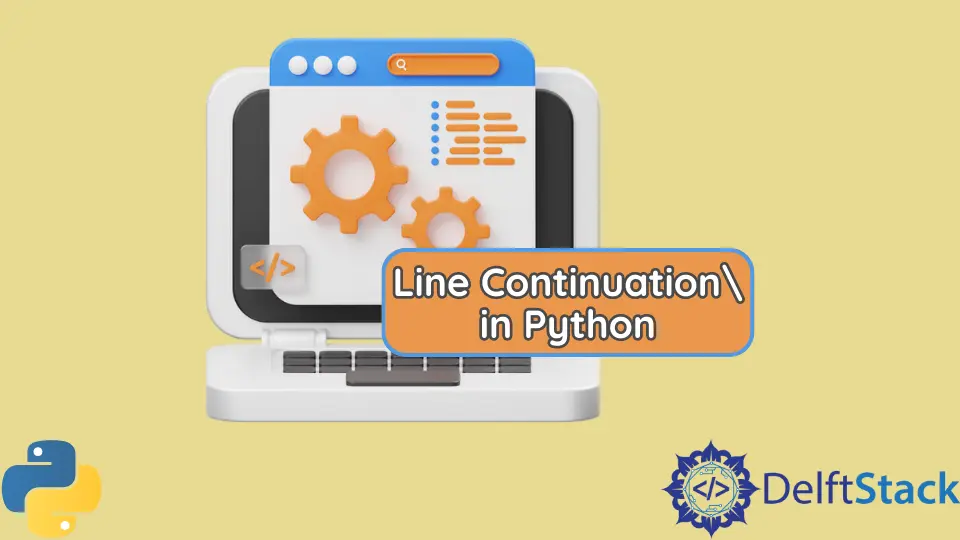
In this tutorial, we will discuss methods for line continuation in Python.
Line Continuation With Explicit Line Break in Python
The \
operator, also known as an explicit line break, can be used to break a single continued long line into many smaller and easy-to-read lines of code. The following code example shows us how we can add a line break for line continuation in Python.
string = (
"This" + " is" + " a" + " string" + " with" + " a" + " double" + " line" + " value"
)
print(string)
Output:
This is a string with a double line value
We broke down a long line of strings into two smaller and easy-to-read lines with an explicit line break in the above code. It can also be done with other types of variables, as shown in the example below.
i = 1 + 2 + 3
x = 1.1 + 2.2 + 3.3
print(i)
print(x)
Output:
6
6.6
The only problem with this approach is that it gives the error SyntaxError: unexpected character after line continuation character
if there is a blank space after the \
.
Line Continuation With ()
in Python
Another method that can be used for line continuation is to enclose the lines inside ()
. The following code example shows us how we can use ()
for line continuation in Python.
string = (
"This" + " is" + " a" + " string" + " with" + " a" + " double" + " line" + " value"
)
print(string)
Output:
This is a string with a double line value
In the above code, we broke down a long line of strings into two smaller and easy-to-read lines by enclosing the lines inside the ()
. This can also be done with other types of variables, as shown in the example below.
i = 1 + 2 + 3
x = 1.1 + 2.2 + 3.3
print(i)
print(x)
Output:
6
6.6
According to the official Python style guide, the ()
approach is much more preferable than the explicit line break.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn