How to Sort With Lambda in Python
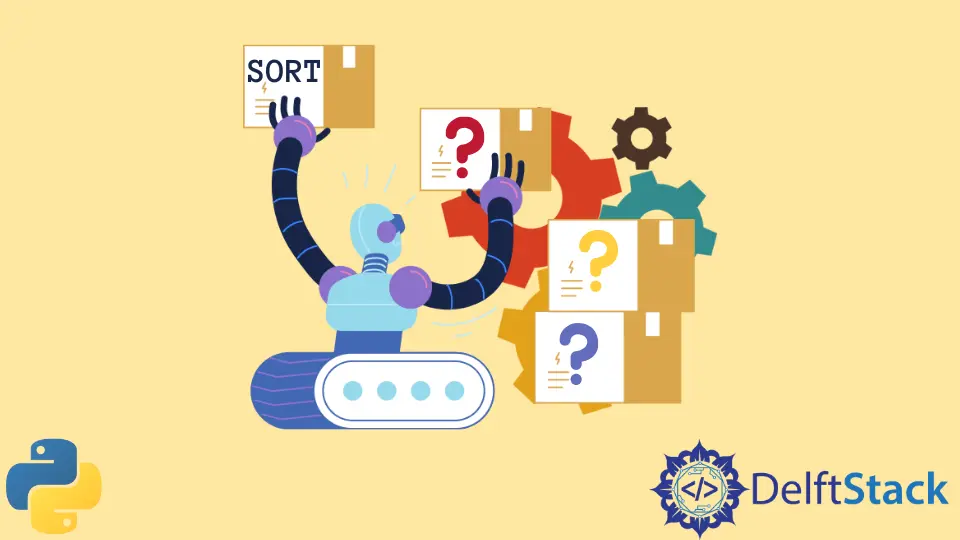
In Python, we have sorted()
and sort()
functions available to sort a list.
These functions allow us to sort the list in the required order. By default, we can sort the list in descending or ascending order.
We can also use one parameter in these functions, which is the key
parameter. It allows us to create our very own sorting order. We can use the lambda
functions in this parameter, enabling us to create our own single-line function.
For example,
lst = ["id01", "id10", "id02", "id12", "id03", "id13"]
lst_sorted = sorted(lst, key=lambda x: int(x[2:]))
print(lst_sorted)
Output:
['id01', 'id02', 'id03', 'id10', 'id12', 'id13']
In the above example, we have a list of IDs where every number is prefixed with the letters id
. In the key
parameter, we specify a lambda
function specifying that we have to ignore the first two characters (id
) and sort the list.
There are also other ways in which we can use the lambda
functions for sorting.
For example,
lst = [("Mark", 1), ("Jack", 5), ("Jake", 7), ("Sam", 3)]
lst_sorted = sorted(lst, key=lambda x: x[1])
print(lst_sorted)
Output:
[('Mark', 1), ('Sam', 3), ('Jack', 5), ('Jake', 7)]
In the above example, we have a list of tuples. The tuple consists of a name and a number. In the lambda
function, we specify the function to sort based on the second element of the tuple, that is, the number.
Note that we can change the order to descending using the reverse
parameter and setting it to True
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn