How to Join Threads in Python
- Understanding the Join Method
- Using Join with Multiple Threads
- Handling Exceptions in Threads with Join
- Conclusion
- FAQ
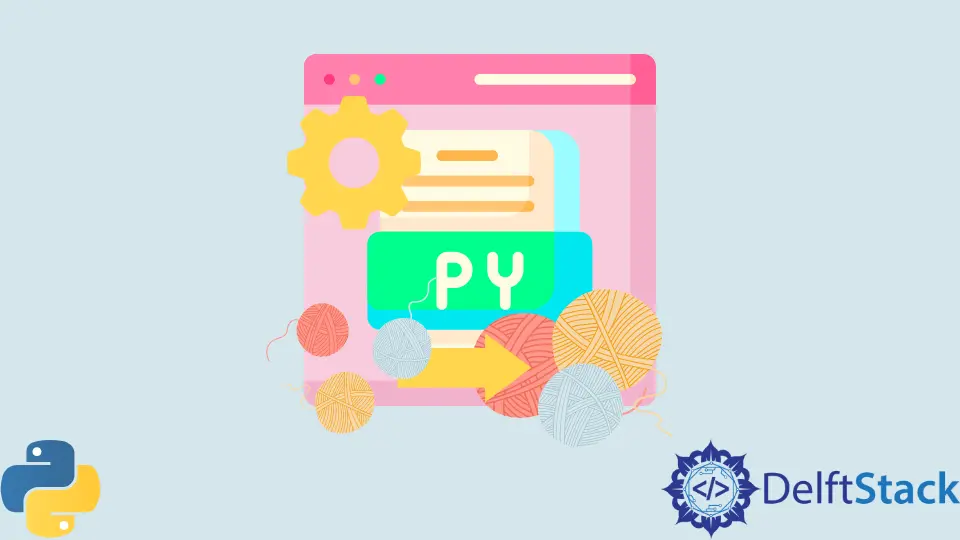
In the world of programming, threading is a powerful technique that allows you to run multiple operations concurrently. Python, with its simple and intuitive syntax, makes it easy to implement threading in your applications. One of the essential methods in threading is the join
method, which ensures that a thread completes its task before the main program continues.
In this tutorial, we’ll delve into how to use the join
method effectively in Python. Whether you’re a beginner or have some experience, you’ll find valuable insights and practical examples that will enhance your understanding of threading in Python. So, let’s get started and unlock the full potential of concurrent programming!
Understanding the Join Method
The join
method is a crucial part of Python’s threading module. When you create a thread, it runs independently of the main program. However, there are times when you need to wait for a thread to finish its execution before proceeding. This is where the join
method comes in. By calling join
on a thread, you essentially pause the execution of the main program until the thread has completed its task.
Here’s a simple example to illustrate how the join
method works in Python:
import threading
import time
def worker():
print("Thread starting...")
time.sleep(2)
print("Thread finished.")
thread = threading.Thread(target=worker)
thread.start()
thread.join()
print("Main program continues...")
In this example, we define a worker
function that simulates a task by sleeping for two seconds. We then create a thread to run this function and start it. The join
method is called on the thread, which means the main program will wait for the thread to finish before printing “Main program continues…”.
Output:
Thread starting...
Thread finished.
Main program continues...
The join
method is beneficial when you need to ensure that certain tasks are completed before moving on. It helps maintain the order of execution, especially when dealing with shared resources.
Using Join with Multiple Threads
When working with multiple threads, the join
method becomes even more vital. You might have several threads performing different tasks, and you may want to wait for all of them to finish before continuing with your program. This can be done using a loop to call join
on each thread.
Let’s take a look at a more complex example that demonstrates how to use join
with multiple threads:
import threading
import time
def worker(thread_number):
print(f"Thread {thread_number} starting...")
time.sleep(1)
print(f"Thread {thread_number} finished.")
threads = []
for i in range(5):
thread = threading.Thread(target=worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All threads have completed.")
In this example, we create five threads, each running the worker
function. Each thread receives a unique number as an argument. After starting all the threads, we loop through the list of threads and call join
on each one. This ensures that the main program waits until all threads have completed their execution.
Output:
Thread 0 starting...
Thread 1 starting...
Thread 2 starting...
Thread 3 starting...
Thread 4 starting...
Thread 0 finished.
Thread 1 finished.
Thread 2 finished.
Thread 3 finished.
Thread 4 finished.
All threads have completed.
Using join
in this way is essential for coordinating the completion of multiple threads. It prevents the main program from terminating before all threads have finished their tasks, ensuring that you have a smooth and predictable execution flow.
Handling Exceptions in Threads with Join
When working with threads, exceptions can occur, and it’s essential to handle them gracefully. The join
method can be used in conjunction with try-except blocks to catch any exceptions that may arise in a thread. This ensures that your main program can respond appropriately without crashing.
Here’s an example that demonstrates how to handle exceptions using join
:
import threading
import time
def worker(thread_number):
if thread_number == 2:
raise Exception("An error occurred in thread 2")
print(f"Thread {thread_number} starting...")
time.sleep(1)
print(f"Thread {thread_number} finished.")
threads = []
exceptions = []
for i in range(5):
thread = threading.Thread(target=worker, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
try:
thread.join()
except Exception as e:
exceptions.append(str(e))
if exceptions:
print("Exceptions occurred:", exceptions)
else:
print("All threads have completed without exceptions.")
In this example, we simulate an error in the worker
function for thread number 2. We create a list to store any exceptions that occur. When we call join
, we wrap it in a try-except block to catch any exceptions. If an exception occurs, we append it to the exceptions
list.
Output:
Thread 0 starting...
Thread 1 starting...
Thread 2 starting...
Thread 3 starting...
Thread 4 starting...
Thread 0 finished.
Thread 1 finished.
Thread 2 finished.
Thread 3 finished.
Thread 4 finished.
Exceptions occurred: ['An error occurred in thread 2']
In this case, the main program can handle the exception gracefully, allowing you to log errors or take corrective action without crashing. This approach is crucial for building robust applications that utilize threading.
Conclusion
In this tutorial, we’ve explored the join
method in Python threading, highlighting its importance in managing thread execution. We’ve examined how to use join
with single and multiple threads, as well as how to handle exceptions gracefully. By mastering the join
method, you can enhance the reliability and efficiency of your Python applications. Whether you’re building a simple script or a complex system, understanding threading is a vital skill in your programming toolkit. Happy coding!
FAQ
-
What is the purpose of the join method in Python threading?
The join method is used to wait for a thread to finish its execution before proceeding with the main program. -
Can I use join with multiple threads?
Yes, you can use join with multiple threads to ensure all threads complete before the main program continues. -
How do I handle exceptions in threads?
You can handle exceptions in threads by using try-except blocks around the join method. -
Is threading in Python suitable for CPU-bound tasks?
Threading is more suitable for I/O-bound tasks due to Python’s Global Interpreter Lock (GIL). For CPU-bound tasks, consider using multiprocessing.
- How can I improve thread performance in Python?
To improve thread performance, minimize shared resources and use synchronization mechanisms like locks when necessary.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn