Java to Python Converter
- Use an External Tool to Translate the Code From Java to Python
-
Use
Jython
Java Library in Python to Translate the Code From Java to Python
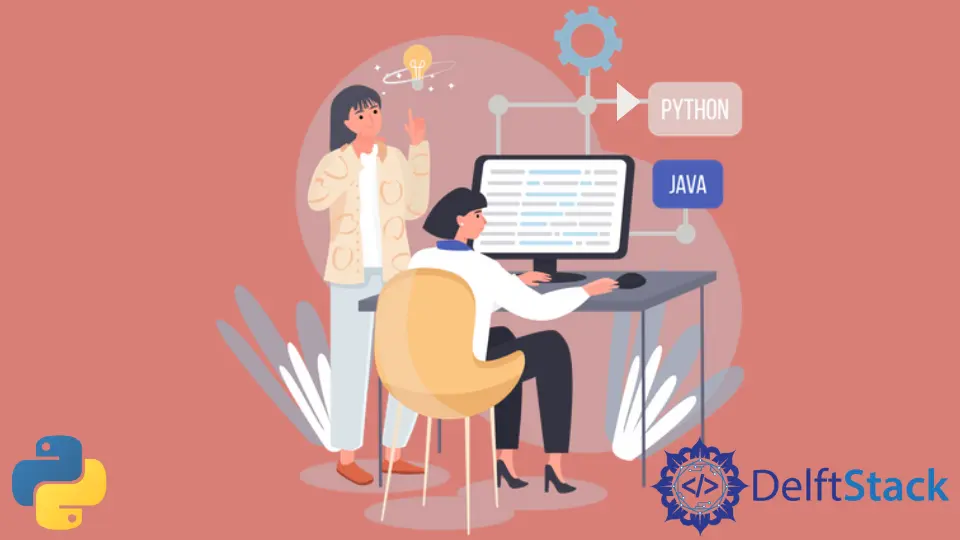
In this tutorial, we will demonstrate the different means by which we can convert Java code to Python code. The process of translation of Java code to Python can be done either manually or with the help of external tools, which have been discussed in the article below.
Use an External Tool to Translate the Code From Java to Python
The tool java2python
can convert a chunk of code written in Java to Python. It swiftly converts the given code to Python and saves ample time for the programmer.
The tool is simple to use and implement and can be directly installed on your device; the instructions are mentioned below for ease of understanding.
-
First, we need to download and extract the
java2python
file with the extensiongzip
. -
The extracted folder contents will be placed on the device’s root folder.
-
Then, the command prompt can be opened and then navigated to
C:\java2python
before utilizing thepython setup.py install
command. -
Afterward, the directory needs to be changed to
C:\java2python\bin
. -
The Java file that needs to be converted is copied to the extracted
java2python
folder. -
The command below is then run on the command line interface. The names
input_file
andoutput_file
indicate the input and output filenames, respectively.j2py -i input_file.java -o output_file.py
-
Finally, the conversion process completes, and the output file contains the code converted to Python.
Using an external tool saves a lot of time, but some data may be lost during an automatic conversion, which makes this method not entirely perfect.
The converted code may not be perfect and might need some edits afterward, but it saves time and hassle if the given Java code is manually translated to Python.
Use Jython
Java Library in Python to Translate the Code From Java to Python
In some cases, translating code from Java to Python might be a target. In contrast, in the other cases, we might need an implementation of Python in Java or vice-versa, which is where the Jython
application comes into play.
The following code is an example of utilizing Java from Python.
from java.lang import System # Java import
print("The Java version Running: " + System.getProperty("java.version"))
print("Java Unix time: " + str(System.currentTimeMillis()))
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn