How to Iterate Through a Tuple in Python
Vaibhav Vaibhav
Feb 02, 2024
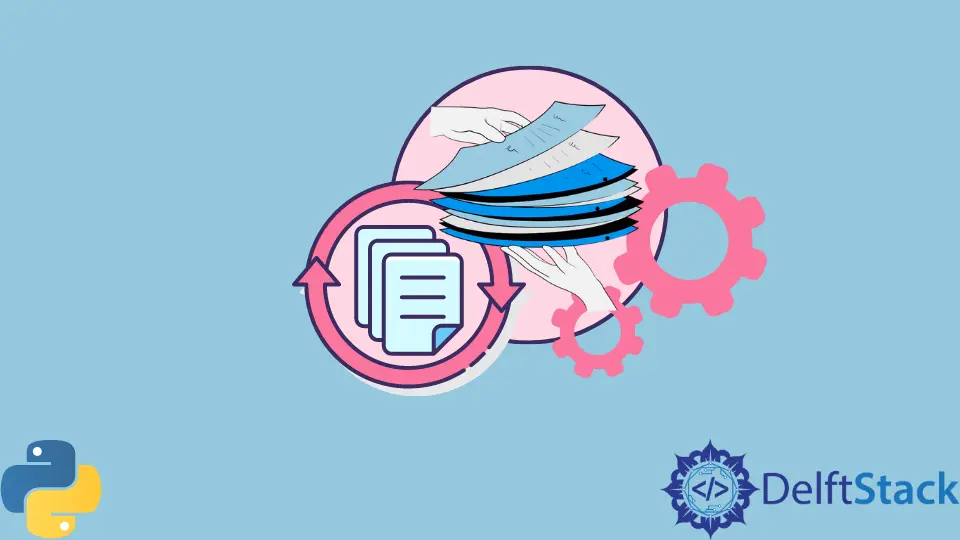
Unpacking in Python refers to assigning values of a list or a tuple to variables using a single line of code. In this article, we will learn how to unpack a tuple in a for
loop using Python.
Unpack a Tuple in a for
Loop in Python
We can use Python’s unpacking syntax to unpack a tuple in a for
loop. The syntax for the unpacking is as follows.
x1, x2, ..., xn = <tuple of length n >
The number of variables on the left side or before the equals sign should equal the length of the tuple or the list. For example, if a tuple has 5
elements, then the code to unpack it would be as follows.
a = tuple([1, 2, 3, 4, 5])
x1, x2, x3, x4, x5 = a
print(x1)
print(x2)
print(x3)
print(x4)
print(x5)
Output:
1
2
3
4
5
We can use the same syntax to unpack values within a for
loop. Refer to the following Python code for the same.
a = tuple(
[("hello", 5), ("world", 25), ("computer", 125), ("science", 625), ("python", 3125)]
)
for x, y in a:
print(f"{x}: {y}")
Output:
hello: 5
world: 25
computer: 125
science: 625
python: 3125
Each value tuple inside the parent tuple is unpacked in variables x
and y
.
Author: Vaibhav Vaibhav