isalpha() in Python
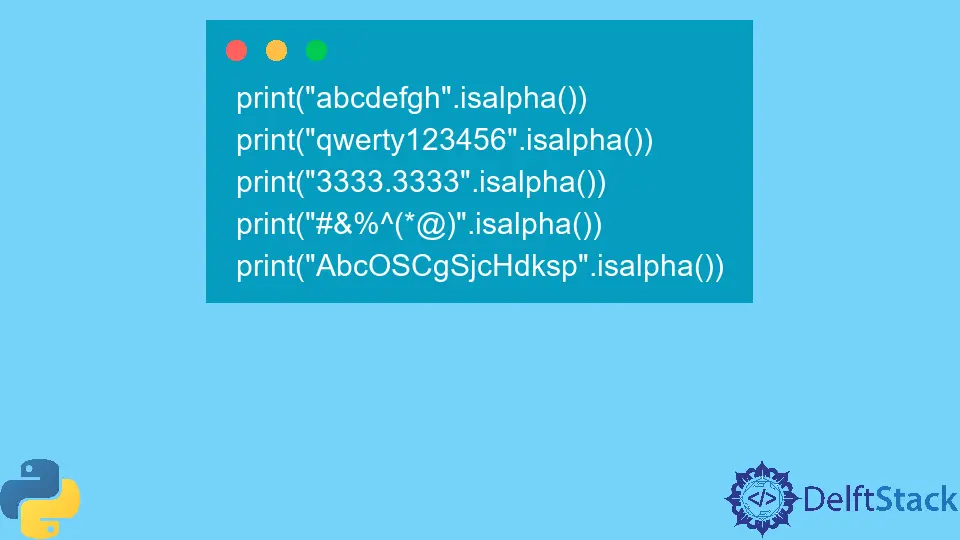
A group of characters joined together to form a string. These characters can be anything; letters such as a
, B
, y
, and Z
, numbers such as 1
, 0
, 9
, and 8
, special characters such as !
, &
, *
and %
.
When working on real-world applications, developers have to validate strings to ensure that the data does not give birth to unexpected bugs. Validation includes cases such as checking for blocklisted characters, checking if the string is uppercase, or if it contains just numbers or not.
Since these tasks are pretty standard, almost all the programming languages own some utility. In this article, we will learn about one such in-built method, isalpha()
in Python.
isalpha()
Method in Python
The isalpha()
method checks if a string is made up of just letters or not.
If it finds any other character, such as a number or a special character, it returns False
. Otherwise, for a valid string, it returns True
.
The isalpha()
method can be called on any string.
Refer to the following Python code for some examples.
print("abcdefgh".isalpha())
print("qwerty123456".isalpha())
print("3333.3333".isalpha())
print("#&%^(*@)".isalpha())
print("AbcOSCgSjcHdksp".isalpha())
Output:
True
False
False
False
True
Following is the explanation for each string.
True
because it contains just letters.False
because it contains numbers too.False
because it contains numbers.False
because it contains special characters.True
because it contains just letters; it does not matter if they are lowercase or uppercase.