How to Fix Invalid Literal for Int() With Base 10 Error in Python
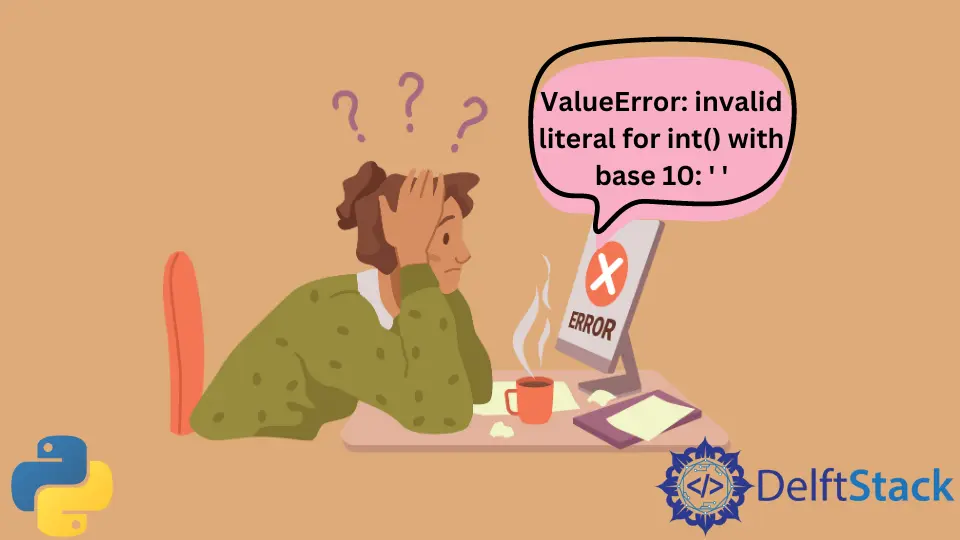
In Python, when converting from one data type to another, we sometimes get the invalid literal for int() with base 10
error. We will learn how to resolve this error and avoid getting it. Let’s dive in.
Fix invalid literal for int() with base 10
Error in Python
This error occurs when converting one data structure into another one. For instance, if we convert some string value to an integer like the following, we get this error because the base of an integer is 10, which differs from other data structures.
# String Value
S1 = "Hello"
# Converting it into integer
number = int(S1)
The above code is incorrect, as we are trying to convert a string value Hello
into an integer that does not make sense. We can’t convert this string value into an integer.
Take a look at another example.
# Other String
S2 = "2.8"
# Converting Float string value in Int
number = int(S2)
In the above code example, the string contains a float value. It will give the error again as it’s the same as converting a string value into an integer with a base of 10. However, this is a float string; there is a way through which you can convert this string into an integer.
# Other String
S2 = "2.8"
# Correct Way to Convert it
# Converting it in to float
F_number = float(S2)
print(F_number)
# Converting Float into int
int_number = int(F_number)
print(int_number)
Output:
2.8
2
First, we will convert it into a float data type. Then we can easily convert that float data type into an integer of base 10.
If a string is an int string, meaning it has an integer value, it will have no problem converting it directly into an integer data type.
# String
S2 = "3"
# Converting string to Int
number = int(S2)
print(number)
Output:
3
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python