How to Fix AttributeError: Int Object Has No Attribute
- Understanding the AttributeError: Int Object Has No Attribute
- Method 1: Check Your Variable Types
- Method 2: Review Your Function Return Values
- Method 3: Use Type Checking
- Conclusion
- FAQ
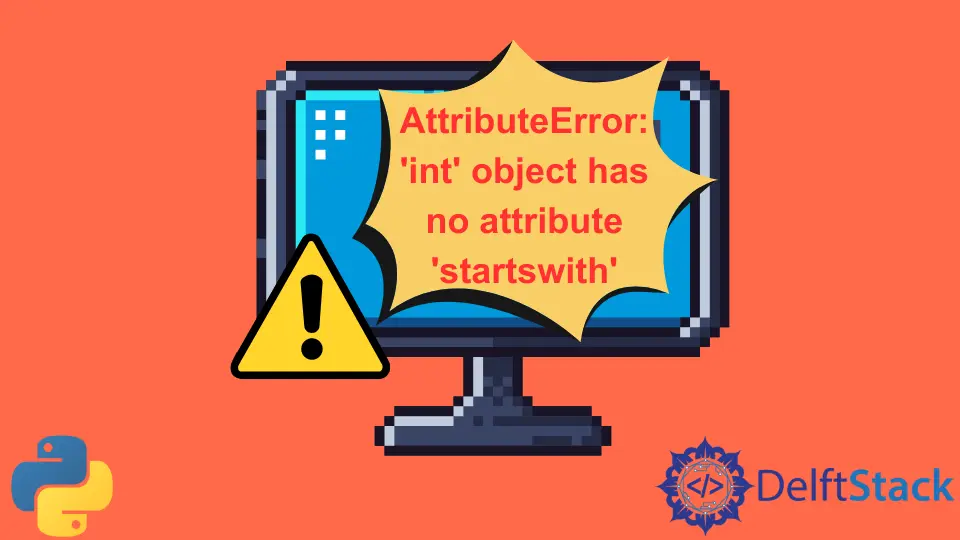
When working with Python, encountering errors is part of the learning process. One common issue developers face is the “AttributeError: ‘int’ object has no attribute” error. This error usually occurs when you’re trying to access an attribute or method that doesn’t exist for an integer object. Understanding why this happens and how to fix it can save you time and frustration.
In this tutorial, we will explore the reasons behind this error and provide practical solutions to help you troubleshoot and resolve it. Whether you are a beginner or an experienced programmer, this guide will equip you with the knowledge needed to tackle this common Python pitfall.
Understanding the AttributeError: Int Object Has No Attribute
Before diving into solutions, it’s essential to understand what this error message means. In Python, every object has a set of attributes and methods. For instance, strings have methods like .upper()
and .lower()
, while lists have methods like .append()
. However, integers (or int
objects) do not possess these attributes. When you attempt to call a method or access an attribute that does not exist for an integer, Python raises an AttributeError.
For example, if you try to call .append()
on an integer, you’ll encounter this error. It’s crucial to check your code for such mistakes. Let’s look at some examples of how this error can manifest and how you can rectify it.
Method 1: Check Your Variable Types
One of the most straightforward ways to resolve the “AttributeError: ‘int’ object has no attribute” issue is to check your variable types. This step ensures that you are working with the correct data types throughout your code.
Here’s a simple snippet that could cause this error:
my_number = 5
my_number.append(10)
When you run this code, you’ll see the following error:
AttributeError: 'int' object has no attribute 'append'
To fix this, you need to ensure that you are using the right data type. Instead of using an integer, you should use a list if you want to use the .append()
method.
Here’s the corrected code:
my_list = [5]
my_list.append(10)
print(my_list)
Output:
[5, 10]
In this corrected version, we first create a list containing the integer 5. We then use the .append()
method to add the integer 10 to the list. This change resolves the AttributeError because lists have the .append()
method, while integers do not.
Method 2: Review Your Function Return Values
Another common source of the AttributeError is returning the wrong type from a function. If a function is expected to return a list but instead returns an integer, you might encounter this error when you try to call a method on the return value.
Consider the following example:
def get_numbers():
return 5
numbers = get_numbers()
numbers.append(10)
Running this code produces the same error:
AttributeError: 'int' object has no attribute 'append'
The function get_numbers()
returns an integer instead of a list. To resolve this, modify the function to return a list:
def get_numbers():
return [5]
numbers = get_numbers()
numbers.append(10)
print(numbers)
Output:
[5, 10]
Now, the function returns a list, allowing you to use the .append()
method without any issues. Always ensure that your functions return the expected data types to avoid such errors.
Method 3: Use Type Checking
In some cases, you might want to ensure that a variable is of the correct type before performing operations on it. Using type checking can help you avoid the AttributeError by allowing you to handle different data types appropriately.
Here’s an example where type checking can prevent the error:
def add_to_list(item, collection):
if isinstance(collection, list):
collection.append(item)
else:
print("The collection must be a list")
my_number = 5
add_to_list(10, my_number)
This code will produce the following error:
AttributeError: 'int' object has no attribute 'append'
To fix this, we can use type checking:
def add_to_list(item, collection):
if isinstance(collection, list):
collection.append(item)
else:
print("The collection must be a list")
my_list = [5]
add_to_list(10, my_list)
print(my_list)
Output:
[5, 10]
By using isinstance()
, we check if collection
is a list before attempting to call .append()
. This way, we avoid the AttributeError and provide a helpful message if the type is incorrect.
Conclusion
The “AttributeError: ‘int’ object has no attribute” error can be frustrating, but it’s a common issue that many Python developers face. By understanding the reasons behind this error and employing the methods discussed in this tutorial, you can troubleshoot and resolve it effectively. Always check your variable types, review your function return values, and consider implementing type checking in your code. With these strategies, you’ll be better equipped to handle similar errors in the future and write more robust Python code.
FAQ
-
What causes the AttributeError: ‘int’ object has no attribute error?
This error occurs when you attempt to access an attribute or method that is not defined for an integer object. -
How can I prevent this error in my code?
You can prevent this error by checking the types of your variables, ensuring that functions return the correct data types, and using type checking before performing operations. -
Can I encounter this error with other data types?
Yes, this error can occur with any data type when you try to access an attribute or method that is not defined for that type. -
Is there a way to debug this error effectively?
Print statements or using a debugger to inspect variable types and values can help you identify the source of the error. -
What should I do if I encounter this error in a library function?
Check the library’s documentation to ensure you are using the function correctly and passing the appropriate data types.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python AttributeError
- How to Fix AttributeError: __Exit__ in Python
- How to Fix AttributeError: 'NoneType' Object Has No Attribute 'Text' in Python
- How to Solve Python AttributeError: _csv.reader Object Has No Attribute Next
- How to Solve Python AttributeError: '_io.TextIOWrapper' Object Has No Attribute 'Split'
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python