How to Install Python 2 and 3 on the Same Device
- Run Python 2 and Python 3 on the Same Device by Renaming the Files
-
Run Python 2.x and Python 3.x on the Same Device Using the
py
Command
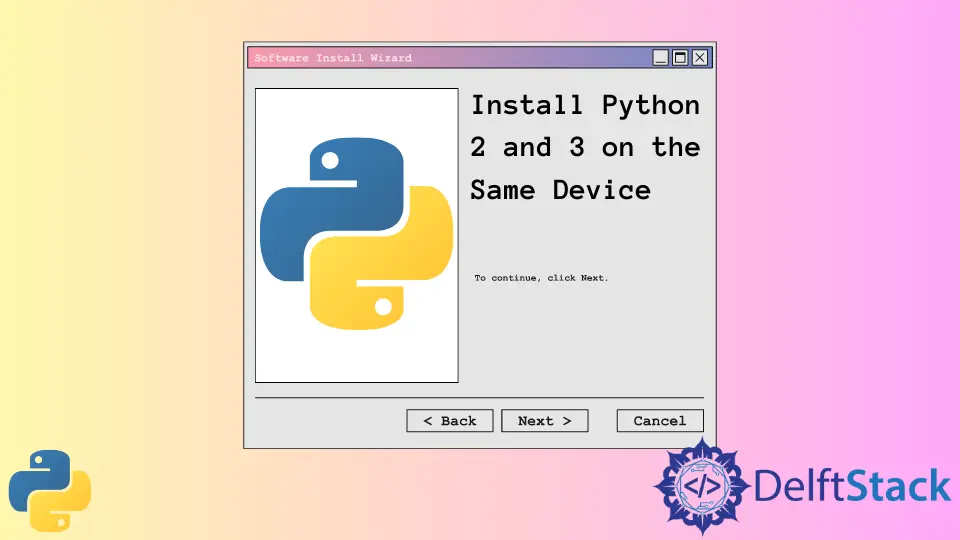
Python is updated with time to the latest standards and with new features. The non-profit organization called Python Software Foundation owns the copyright and manages the language updates for Python 2.1 and above.
The two most commonly used versions of Python are Python 2.x and Python 3.x. A few significant differences exist between the two, so sometimes a script compatible in Python 2.x might not run in Python 3.x and vice-versa.
We can have both Python 2 and Python 3 installed on any Windows or Linux device. We can either create different environments on different IDEs to use the versions separately or use the following ways to run them using the command prompt.
Run Python 2 and Python 3 on the Same Device by Renaming the Files
To obtain Python 2.x and Python 3.x on the same machine, you need to follow the following steps.
-
Install Python 2.x and Python 3.x with the default windows installers.
-
Go to the default installation path (
C:\Python3x
) and renamepython.exe
topython3.exe
. -
Edit your environment variables to include the following directory link
C:\Python27\;C:\Python27\Scripts\;C:\Python34\;C:\Python34\Scripts\
The above is based on installing Python 2.7 and Python 3.4. Edit the versions based on your requirements.
Note that there can be a conflict when you change your environment variables because the two versions have the same name: python.exe
.
Therefore, if you run through this error, ensure that you have followed step 2 carefully and renamed your python.exe file to python3.exe
. So when the user runs python
, version 2.x will get executed. With python3
, the 3.x version will get executed, giving you access to both Python 2 and Python 3 on the same device.
Run Python 2.x and Python 3.x on the Same Device Using the py
Command
We can use the py
command to launch different versions of Python on the same device. Note the steps below.
-
Install the required version of Python 2
-
Install the required version of Python 3
-
Open the command prompt on your device.
-
In the command prompt, type
py -2.x
to launch any version of Python 2. -
In the command prompt, type
py -3.x
to launch any version of Python 3.