Inline if...else Statement in Python
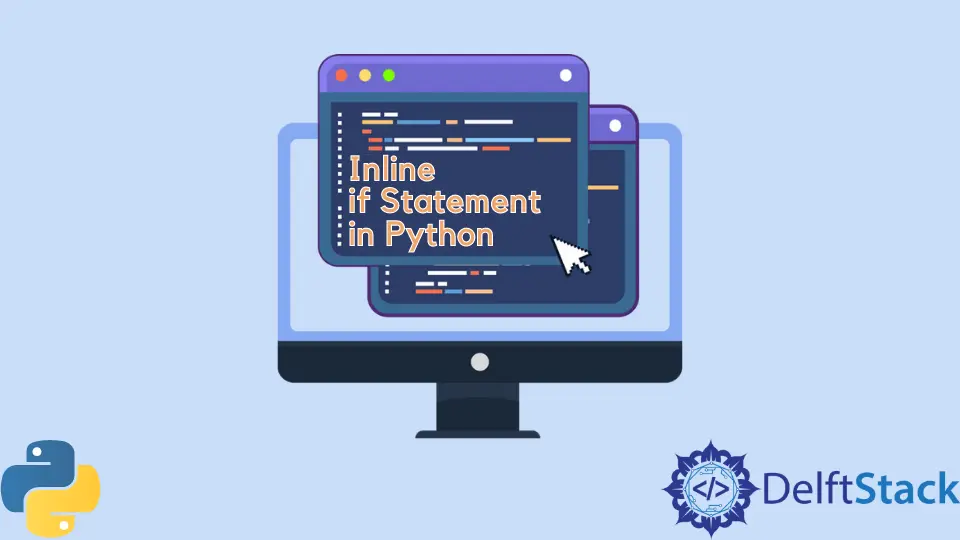
The if ... else
statement is frequently used for evaluating conditions in many programming languages.
Python has an inline if ... else
statement, which allows a compact version of the if ... else
statement in a single line. Such an inline statement is restricted and can only contain multiple if ... else
if they are carefully cascaded. However, they must contain the else
clause; otherwise, it won’t work.
Such statements improve the readability of the code, make it shorter and cleaner, and can be used while assigning values or other functions.
In the code below, we will use it while assigning some value to a variable based on another variable’s value.
b = 5
a = 1 if b > 2 else 2
print(a)
Output:
1
Note that due to their similarity, such inline if ... else
statements are considered the ternary operator in Python.
We can also use the inline if ... else
statement with other functions like the print()
to display something based on a condition.
a = 0
b = 2
print(a if a != 0 else b)
Output:
2
We can map multiple if ... else
conditions in inline statements. For example:
b = 5
a = 1 if b == 2 else (2 if b > 3 else 3)
print(a)
Output:
2
The elif
condition cannot be used in inline if ... else
statement.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn