How to Fix ImportError: No Module Named mysql.connector
-
Install MySQL Connector to Fix
ImportError: No module named mysql.connector
in Python -
Import the
mysql.connector
Module in Python
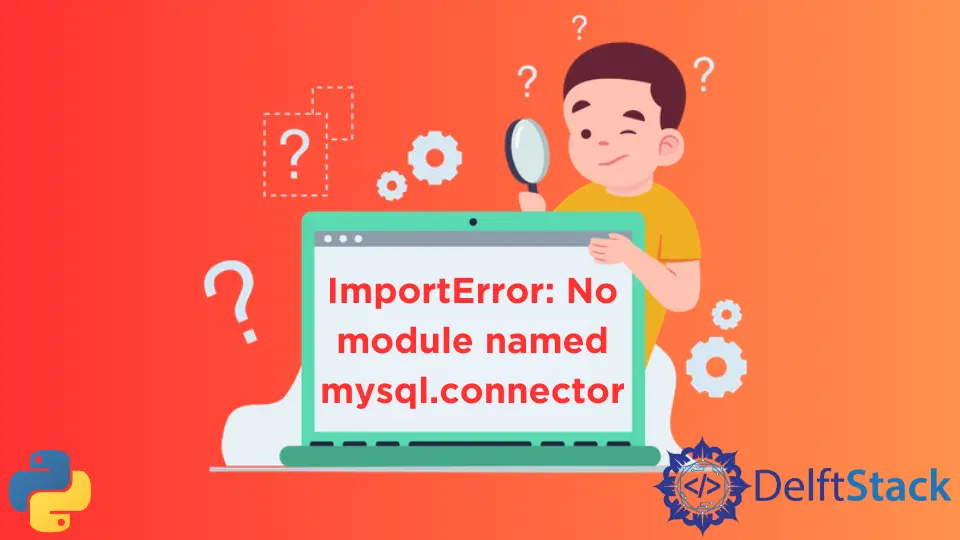
The ImportError
is raised when the Python program cannot import the specified module or member of a module. It is because Python cannot locate the module you are trying to import.
The main reason for this error is that the module is not installed. The ImportError: No module named mysql.connector
can be solved by installing the module MySQL Connector.
This tutorial teaches you to fix the error ImportError: No module named mysql.connector
in Python.
Install MySQL Connector to Fix ImportError: No module named mysql.connector
in Python
The module MySQL Connector does not come with the Python Standard Library. To import the module mysql.connector
, you must install the MySQL driver mysql-connector-python
.
Run the following command in the terminal.
pip install mysql-connector-python
For Python 3, use the command below.
pip3 install mysql-connector-python
You can also install the MySQL driver mysql-connector-python-rf
to import mysql.connector
in Python.
pip install mysql-connector-python-rf
After installing the module, run the Python program again, and the ImportError
must be solved now.
Import the mysql.connector
Module in Python
Verify if the installation was successful by importing the module mysql.connector
.
Run the import command in Python.
import mysql.connector
The MySQL Connector is successfully installed if it does not return any errors.
Let’s see an example of a Python program to connect to the MySQL server.
import mysql.connector
cnx = mysql.connector.connect(user="rohan", password="pass1234", host="localhost")
print(cnx)
The connect()
constructor helps to establish a connection to the MySQL server. Replace the user, password, and host to match values in your MySQL server.
Output:
<mysql.connector.connection.MySQLConnection object at 0x000001B6F8BE0D30>
It returns a MySQLConnection
object.
Now you know how to fix the ImportError: No module named mysql.connector
in Python. You have learned to install the MySQL Connector and create a connection to the MySQL server.
Related Article - Python ImportError
- How to Fix ImportError: Missing Required Dependencies Numpy
- How to Fix ImportError: No Module Named Sklearn in Python
- How to Fix Python ImportError: No Module Named Requests
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python