How to Fix ImportError: Cannot Import Name _Remove_dead_weakref in Python
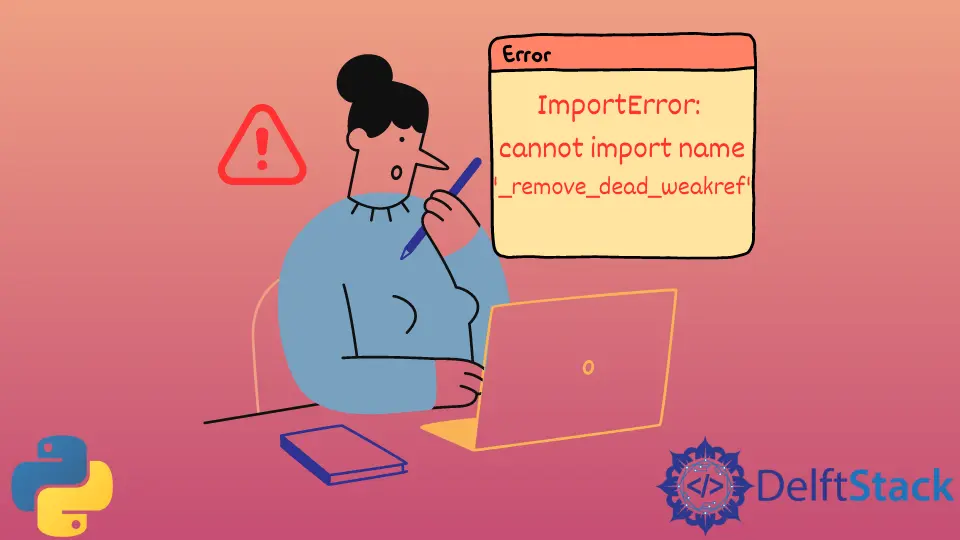
In Python, we use modules to define and store functionalities and classes in files, which can be imported into other programs. We use the import
statement to import the functions and objects from a module.
ImportError: cannot import name _remove_dead_weakref
in Python
The ImportError
is an exception that occurs while importing some modules. This can be because the module does not exist or due to another fault.
This tutorial will discuss the ImportError: cannot import name _remove_dead_weakref
error in Python and ways to fix it.
Sometimes a module can import other modules if specified in the module, including some standard modules like sys
, weakref
, os
, and more. The weakref
module is associated with creating weak references to some objects that help in garbage collection and in freeing up space.
The ImportError: cannot import name _remove_dead_weakref
is not directly associated with any problem in the module but can happen if multiple versions of Python are installed on the device incorrectly.
Multiple versions of Python can exist on a device, but one needs to do this carefully. This error is caused if some Python version is installed by copying the installation folder, renaming previous directories, or setting the wrong Path
in the Environment Variables.
To fix this, ensure that Python is installed at the desired location and set the user permissions accordingly. One can also remove the undesirable version of Python.
We can also use virtual environments to handle multiple versions of Python simultaneously. We can also use pyenv
, Anaconda, PyCharm, and other IDEs.
A virtual environment is very useful as it isolates the given modules and Python from other environments, so there is no internal conflict.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python