How to Import a File in Python
-
Import a File With the
import
Statement in Python -
Import a File With the
importlib
Module in Python -
Import a Specific Module From a File With the
from
Clause in Python
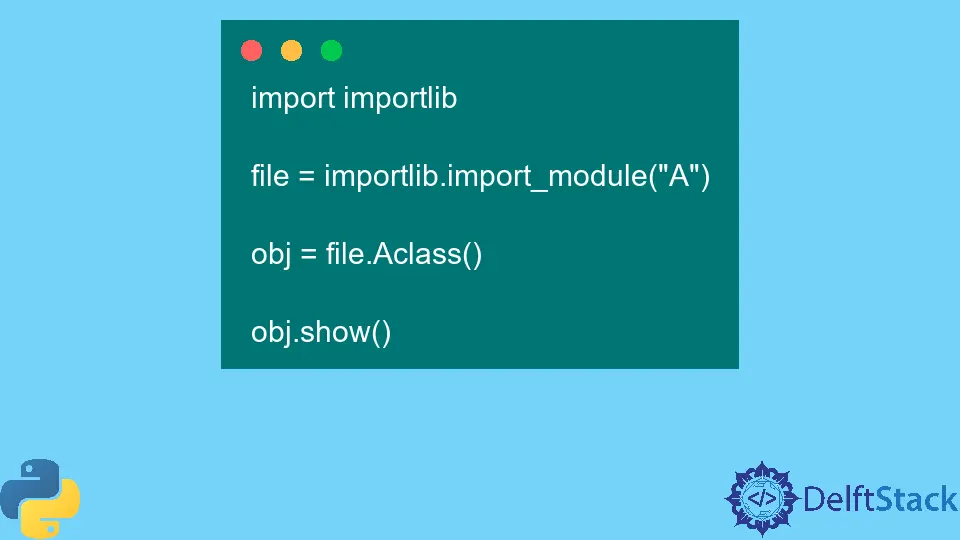
In this tutorial, we will discuss methods to import a file in Python.
Import a File With the import
Statement in Python
The import
statement is used to import packages, modules, and libraries in Python. The import
statement can also be used to import files. For this tutorial, we have two code files, A.py
and main.py
. The contents of the A.py
code files are given below.
A.py
file:
class Aclass:
a = 5
def show(this):
print("Hello! this is class A")
We want to import this A.py
file code in our main.py
file. The following code example shows us how we can import files into our code with the import
statement in Python.
main.py
file:
import A
obj = A.Aclass()
obj.show()
Output:
Hello! this is class A
In the above code, we import the A.py
file and call the show()
function inside the Aclass
class.
Import a File With the importlib
Module in Python
The importlib
module has many methods for interacting with the import system of Python. The importlib.import_module()
function can be used to import files inside our code. The following code example shows us how we can import files into our code with the importlib
module in Python.
import importlib
file = importlib.import_module("A")
obj = file.Aclass()
obj.show()
Output:
Hello! this is class A
In the above code, we imported the A.py
file code with the importlib
module and called the show()
function inside the Aclass
class.
Import a Specific Module From a File With the from
Clause in Python
The from
clause can be added to the conventional import
statement to import only a subset of the file in Python. The from
clause is useful if we only want to import one or more modules from a file but not the complete file itself. The following code example shows us how to import a specific module from a file into our code with the from
clause in Python.
from A import Aclass
var1 = Aclass()
var1.show()
Output:
Hello! this is class A
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn