if Statement With Strings in Python
- Basic String Comparison
-
Using
in
Keyword with Strings - Case-Insensitive String Comparison
- Advanced String Comparisons
- Conclusion
- FAQs
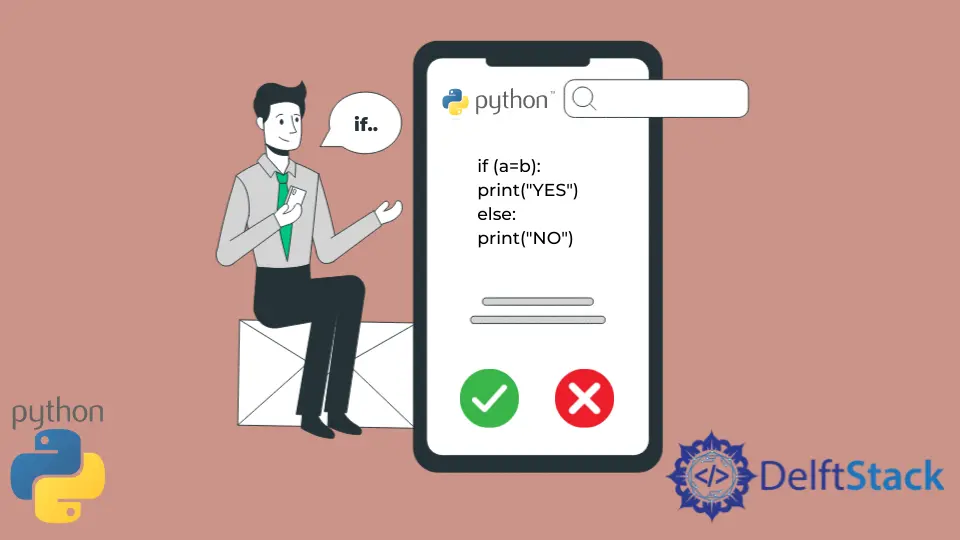
In Python, the if
statement is a fundamental control structure that allows programmers to make decisions in their code. When combined with string manipulation, it becomes a powerful tool for text processing, data validation, and user interaction. This guide will explore various techniques for using if
statements with strings in Python, covering both basic and advanced concepts.
Understanding how to effectively use if
statements with strings is crucial for several reasons:
- It allows you to create more interactive and responsive programs.
- It enables you to process and analyze text data more efficiently.
- It helps in implementing logic for user input validation and decision-making based on textual information.
Let’s dive into the various aspects of using if
statements with strings in Python.
Basic String Comparison
At its core, string comparison in Python involves checking whether two strings are equal or different. This is often the first step in many text-processing tasks. For a more detailed explanation of string equality, check out Python String Comparison.
pythonname = "Alice"
if name == "Alice":
print("Hello, Alice!")
else:
print("You're not Alice.")
In this example, we’re using the equality operator (==
) to compare the value of the name
variable with the string “Alice”. It’s important to note that Python string comparisons are case-sensitive. This means “Alice” and “alice” would be considered different strings.
We can also compare string lengths, which is useful when you need to check if a string meets certain length criteria:
pythonword1 = "Python"
word2 = "Programming"
if len(word1) < len(word2):
print(f"{word1} is shorter than {word2}")
else:
print(f"{word1} is not shorter than {word2}")
Here, we’re using the len()
function to get the length of each string and then comparing these lengths. This technique is particularly useful when you need to validate input lengths or compare the sizes of different text elements.
Using in
Keyword with Strings
The in
keyword in Python is versatile and particularly useful when working with strings. It allows you to check if a substring exists within a larger string or if a string is part of a collection of strings. If you need to find multiple occurrences of a character inside a string, refer to Find All Occurrences of a Character in a String.
pythonsentence = "Python is awesome"
if "awesome" in sentence:
print("The sentence contains 'awesome'")
In this example, we’re checking if the word “awesome” is present in the sentence
string. This technique is invaluable when you need to search for specific words or phrases within larger text blocks.
The in
keyword can also be used with lists of strings, which is often useful for input validation:
pythonuser_input = "y"
if user_input.lower() in ["y", "yes", "yeah"]:
print("User agreed")
else:
print("User did not agree")
Here, we’re checking if the user’s input (converted to lowercase) matches any of the affirmative responses in our list. This approach allows for flexible input handling, accommodating various ways a user might express agreement. For an in-depth guide on checking substring presence in Python, read Python Check If String Contains Another String.
Case-Insensitive String Comparison
Often, you’ll want to compare strings regardless of their case. Python doesn’t have a built-in case-insensitive comparison operator, but you can easily achieve this by converting strings to a common case before comparison.
pythonuser_input = "YES"
if user_input.lower() == "yes":
print("Affirmative response received")
In this example, we convert the user’s input to lowercase before comparing it to “yes”. This ensures that “YES”, “yes”, “Yes”, and any other case variation will all be recognized as affirmative responses.
You can also use this technique with the in
keyword for more flexible comparisons:
pythoncolor = "RED"
if color.lower() in ["red", "blue", "green"]:
print("Primary color detected")
else:
print("Not a primary color")
This code checks if the input color (regardless of its case) is one of the primary colors. By converting to lowercase before the check, we ensure case-insensitive comparison.
Use lower()
or upper()
methods to normalize string case for consistent comparisons. To learn more about string case conversions, check out Convert String to Lowercase or Uppercase in Python.
Advanced String Comparisons
Python offers several advanced techniques for string comparison and manipulation that can be used with if
statements.
Anagram Detection
You can use the sorted()
function to check if two words are anagrams (words with the same letters in a different order):
pythonword1 = "listen"
word2 = "silent"
if sorted(word1) == sorted(word2):
print("The words are anagrams")
This code sorts the characters in both words and then compares the sorted versions. If they’re equal, the words are anagrams.
Using String Methods
Python’s string methods provide powerful tools for string manipulation and comparison:
pythonfilename = "document.txt"
if filename.endswith(".txt"):
print("Text file detected")
The endswith()
method checks if a string ends with a specific substring. This is particularly useful for tasks like file type detection.
Multiple Conditions
You can combine multiple string checks using logical operators:
pythonname = "John Doe"
if name.startswith("John") and " " in name:
print("Valid name format")
This code checks if the name starts with “John” and contains a space, which could be a simple validation for a full name format.
Identity Comparison
The is
operator checks if two variables refer to the same object in memory:
pythona = "Python"
b = "Python"
if a is b:
print("a and b refer to the same string object")
While this usually works for short strings due to Python’s string interning, it’s generally recommended to use ==
for string content comparison.
Conclusion
Mastering the use of if
statements with strings in Python opens up a world of possibilities for text processing and decision-making in your code. From basic comparisons to advanced techniques, the flexibility of Python’s string handling makes it an excellent language for working with text data.
Remember these key points:
- String comparisons are case-sensitive by default.
- Use
lower()
orupper()
for case-insensitive comparisons. - The
in
keyword is versatile for substring and list membership checks. - Combine string methods with
if
statements for powerful text processing. - Always consider the specific needs of your program when choosing a comparison technique.
By applying these concepts and techniques, you’ll be well-equipped to handle a wide range of string-related tasks in your Python programs.
FAQs
- Q: Is string comparison in Python case-sensitive?
A: Yes, by default, string comparisons in Python are case-sensitive. Uselower()
orupper()
methods for case-insensitive comparisons. - Q: Can I use
if
statements to check for multiple string conditions?
A: Absolutely! You can use logical operators likeand
,or
, andnot
to combine multiple conditions in anif
statement. - Q: What’s the difference between
==
andis
when comparing strings?
A:==
compares the content of strings, whileis
checks if two variables refer to the same object in memory. For string comparisons,==
is generally preferred. - Q: How can I check if a string starts or ends with specific characters?
A: Use thestartswith()
andendswith()
methods, e.g.,if string.startswith("Hello")
. - Q: Can I use regular expressions in
if
statements for string matching?
A: Yes, you can use there
module to perform regex matching withinif
statements for more complex pattern matching. This is particularly useful for advanced text processing tasks.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python