if not Statement in Python
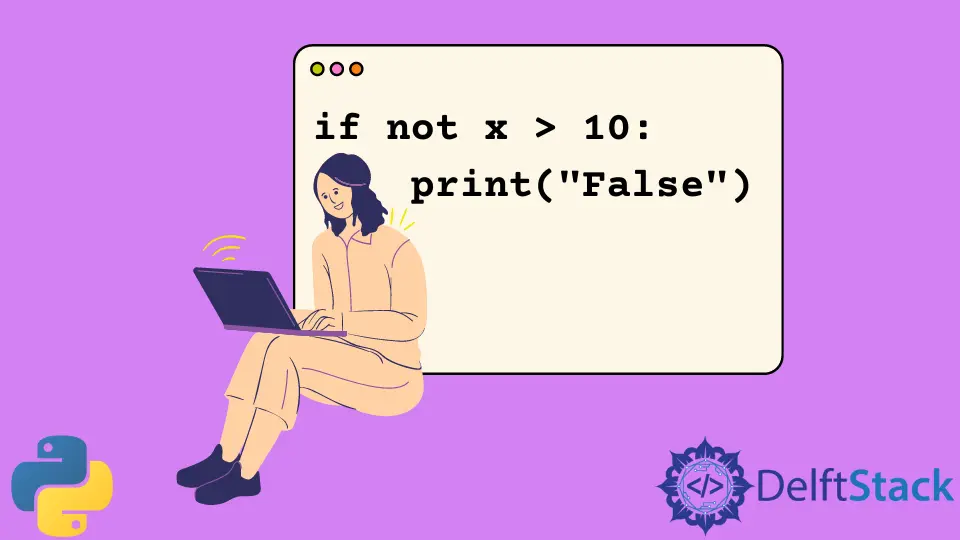
The if
statement in Python checks a specific condition and executes a code block if the condition is true.
The if not
does the opposite of the if
statement. It tests if a condition is false and then execute some statements.
The use of the if not
statement improves the readability of the code and can directly execute some statements for conditions returning False
.
The following code will help in explaining its use.
x = 5
if not x > 10:
print("False")
Output:
False
Since x > 10
is False
, the code gets executed.
Similar to the if
statement, it can have multiple conditions, and we can also use it with the else
keyword to create if-else
blocks.
The if not
statement can also be used to check if a data collection like a list, a dictionary is empty or not. In Python, if a variable or object is 0 or empty, then it is considered false. See the following example.
lst = []
if not lst:
print("Empty")
Output:
Empty
Similarly, its use can extend to other conditions like checking if something is not present in a collection. For example, we can use the if not
with the in
keyword to execute some statements if an element is not present in a list, as shown below.
lst = [1, 2, 3, 5, 6]
if not 4 in lst:
print("All Okay")
else:
print("Not Okay")
Output:
All Okay
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn