if...else in One Line Python
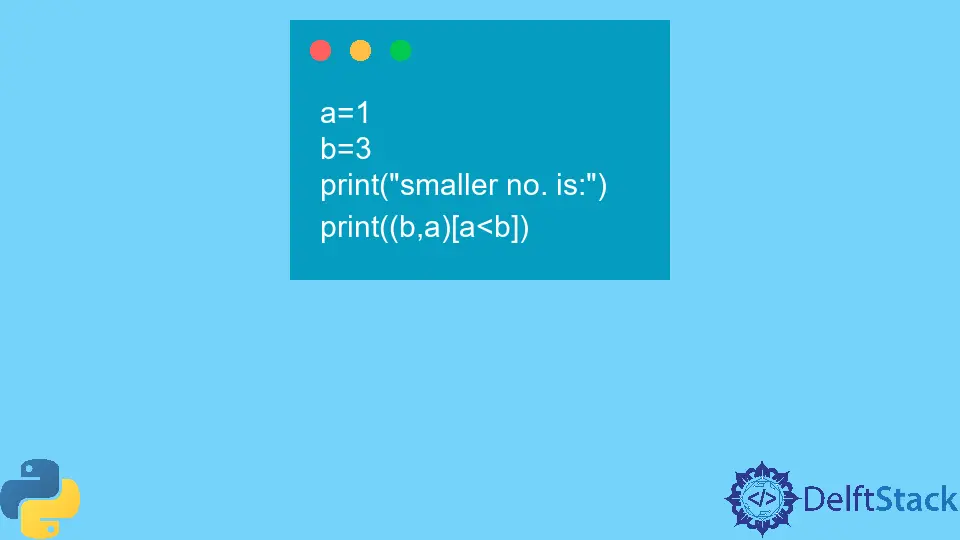
The if-else
statement works as, if the condition is true, then the statement following if
will be executed otherwise, the else
statement will be executed.
The if-else
statement usually takes up to 4 lines of code to its working. Still, sometimes, in return statements and other nested functions, it must be compressed or condensed to make it readable and handy.
This tutorial will help you to condense the if-else
statement into a simple one-line statement in Python.
[if_true] if [expression] else [if_false]
This is the compressed or condensed form of the if-else
statement. In this, [if_true]
is the statement that will be executed if the expression stands true, and if it is false, then the [if_false]
will be executed.
For example,
i = 1
j = 5
min = i if i < j else j
print(min)
Output:
1
There are several alternatives to the condensed if-else
statements. We can also use a tuple method that works similarly.
Syntax for Direct Method using Tuples:
(If_false, if_true)[test_expression]
This tuple method takes two expressions in the form of a tuple against the text expression. If the expression stands false, the first expression will be executed, and if not, then the second expression will be executed.
For example,
a = 1
b = 3
print("smaller no. is:")
print((b, a)[a < b])
Output:
smaller no. is:1
We can also use a dictionary to obtain the same result.
Syntax for Direct Method using Dictionary:
({True
i, False: j}[i < j])
In this, usage of Dictionary is done for selecting an item.
For example,
i = 1
j = 2
print({True: i, False: j}[i < j])
Output:
1