How to Read Input From stdin in Python
-
Use
fileinput.input()
to Read Fromstdin
in Python -
Use
sys.stdin
to Read Fromstdin
in Python - Conclusion
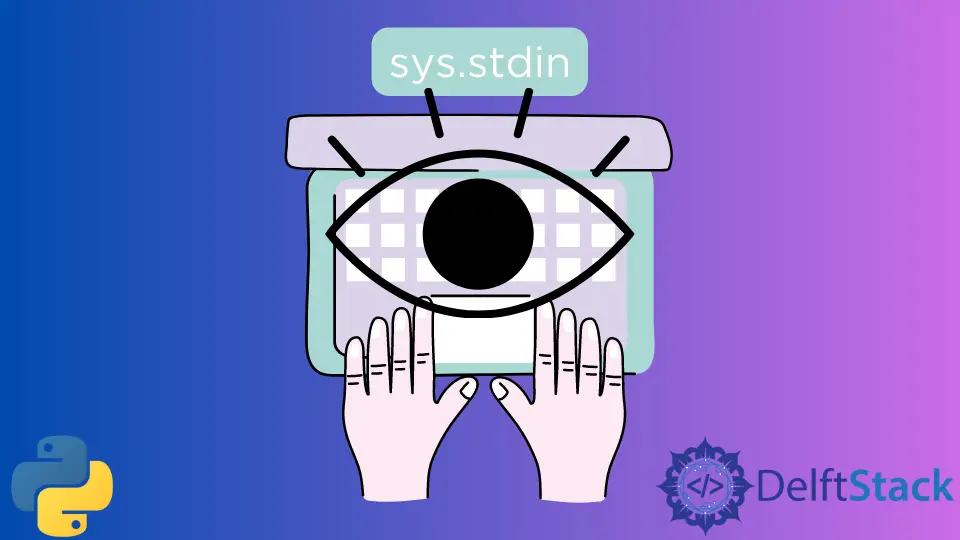
This tutorial discusses methods to read input from stdin
in Python. This can be either reading from the console directly or reading from the filename specified in the console.
Use fileinput.input()
to Read From stdin
in Python
We can use the fileinput
module to read from stdin
in Python. fileinput.input()
reads through all the lines in the input file names specified in command-line arguments. If no argument is specified, it will read the standard input provided.
The below example illustrates reading from the input file name specified.
We will use the sample.txt
below.
Hello
Line1
Line2
Below the script read.py
.
import fileinput
for line in fileinput.input():
print(line.rstrip())
We execute it like this:
python read.py "sample.txt"
Output:
Hello
Line1
Line2
The below example illustrates reading from standard input.
import fileinput
for line in fileinput.input():
print("Output:", line.rstrip())
The execution and output is shown below.
python read.py
Line 1
Output: Line 1
Line 2
Output: Line2
^Z
We can save the data to be processed later as well like this:
import fileinput
data = []
for line in fileinput.input():
data.append(line.rstrip())
Note that we are using line.rstrip()
. That is to remove the trailing newline.
Typing in y
deletes all the variables.
Use sys.stdin
to Read From stdin
in Python
Another approach is to use sys.stdin
to read from stdin
in Python. The below example illustrates reading data from stdin
line by line:
import sys
for line in sys.stdin:
print("Output:", line.rstrip())
The execution and output is shown below.
python read.py
Line 1
Output: Line 1
Line 2
Output: Line2
^Z
We can also read all the data from stdin
in one go instead of line by line.
The below example illustrates this:
import sys
data = sys.stdin.readlines()
data = [line.rstrip() for line in data]
Note that we are using line.rstrip()
. That is to remove the trailing newline.
Conclusion
We discussed 2 methods, fileinput.input()
and sys.stdin
, to read stdin
input in Python. fileinput.input()
can read data from the specified file name in the command line argument or from the standard input, while sys.stdin
can only read data from the standard input.