How to Read User Input as Integers in Python
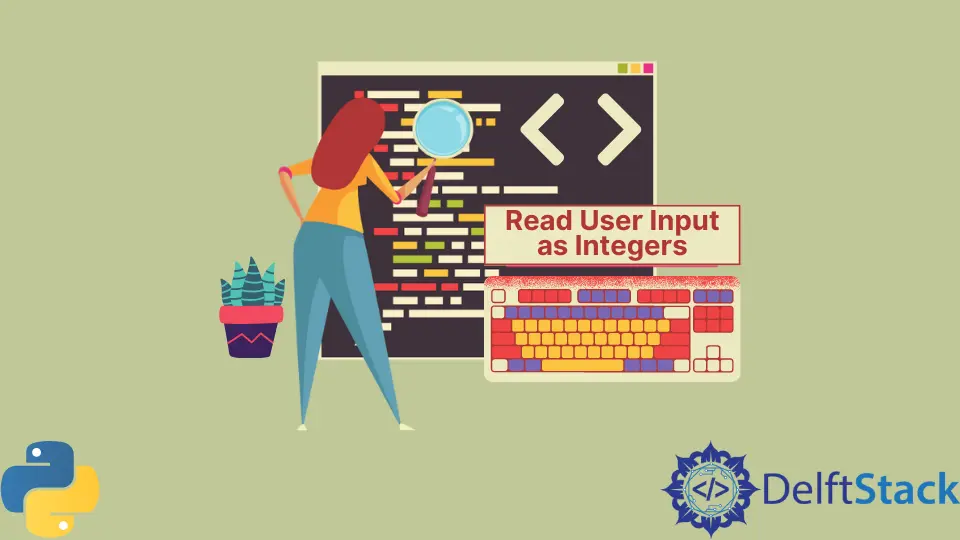
In this tutorial, we will learn how to read user input as integers in Python.
Read User Input as Integers in Python 2.x
Python 2.7 has two functions to read the user input, that are raw_input
and input
.
raw_input
reads the user input as a raw string and its return value is simply string
.
input
gets the user input and then evaluates the string and the result of the evaluation is returned.
For example,
>>> number = raw_input("Enter a number: ")
Enter a number: 1 + 1
>>> number, type(number)
('1 + 1', <type 'str'>)
>>> number = input("Enter a number: ")
Enter a number: 1 + 1
>>> number, type(number)
(2, <type 'int'>)
Consider twice when you use input
in Python 2.x. It could impose security issues because input
evaluates whatever user types.
Let’s say if you have already imported os
in your program and then it asks for user input,
>>> number = input("Enter a number: ")
Enter a number: os.remove(*.*)
Your input os.remove(*.*)
is evaluated and it deletes all the files in your working dictionary without any notice!
Read User Input as Integers in Python 3.x
raw_input
is obsolete in Python 3.x and it is replaced with input
in Python 3.x. It only gets the user input string but doesn’t evaluate the string because of the security risk as described above.
Therefore, you have to explicitly convert the user input from the string to integers.
>>> number = int(input("Enter a number: "))
Enter a number: 123
>>> number
123
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook