How to Read CSV to List in Python
-
Read CSV Into a List in Python Using
csv.reader
-
Read CSV Into a List in Python Using
csv.reader
With Other Delimiters
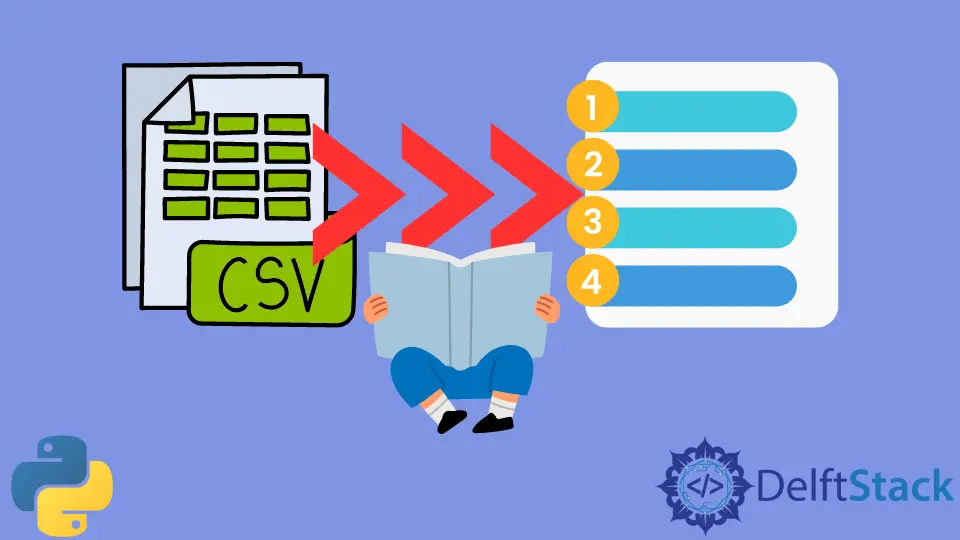
This article introduces how to read CSV to list in Python.
Let’s suppose we have a CSV
file Employees.csv
with the following content in it,
Id | Name | Department | Salary | |
---|---|---|---|---|
1 | Sam | Human Resource | sam@gmail.com | 65K |
2 | John | Management | john@gmail.com | 58K |
3 | Tony | IT | tony@gmail.com | 70K |
4 | Mike | Accounts | mike@gmail.com | 35K |
If you open this file using some text editor, its content should look like this.
Id,Name,Department,email,Salary
1,Sam,Human Resource,sam@gmail.com,65K
2,John,Management,john@gmail.com,58K
3,Tonny,IT,tonny@gmail.com,70K
4,Mike,Accounts,mike@gmail.com,35K
Now we will import the above data of this CSV file into a Python list.
Read CSV Into a List in Python Using csv.reader
Python has a built-in module named CSV
, which has a reader class to read the contents of a CSV file. The example code to read the CSV to a list in Python is as follows.
from csv import reader
with open("Employees.csv", "r") as csv_file:
csv_reader = reader(csv_file)
# Passing the cav_reader object to list() to get a list of lists
list_of_rows = list(csv_reader)
print(list_of_rows)
csv_reader = reader(csv_file)
passes the file ojbect csv_file
to the csv.reader()
function and gets the reader
object. It returns an iterator, which is used to iterate over all the lines of the CSV file.
list_of_rows = list(csv_reader)
converts the csv.reader
object to a list of lists, where each element of the list means a row of CSV, and each item in the list element represents a cell or column in a row.
Output:
[
["Id", "Name", "Company", "email", "Salary"],
["1", "Sam", "Human Resource", "sam@gmail.com", "65K"],
["2", "John", "Management", "john@gmail.com", "58K"],
["3", "Tonny", "IT", "tonny@gmail.com", "70K"],
["4", "Mike", "Accounts", "mike@gmail.com", "35K"],
]
Read CSV Into a List in Python Using csv.reader
With Other Delimiters
The csv.reader
function also provides an option to read the text files in which values are separated by some other character rather than a comma. For example, the delimiter could be a tab or white space. To read such files, we need to pass an extra parameter delimiter
to the reader function. See the example below.
If we have a file Employees_TSV.csv
with the same content as in Employees.csv but separated by tab rather than a comma.
from csv import reader
with open("Employees_TSV.csv", "r") as csv_file:
csv_reader = reader(csv_file, delimiter="\t")
list_of_rows = list(csv_reader)
print(list_of_rows)
We read data from a tab-separated values file in the above code. delimiter = '\t'
specifies that the delimiter in the CSV file is the tab.
The output of this code is the same as the above.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python